Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial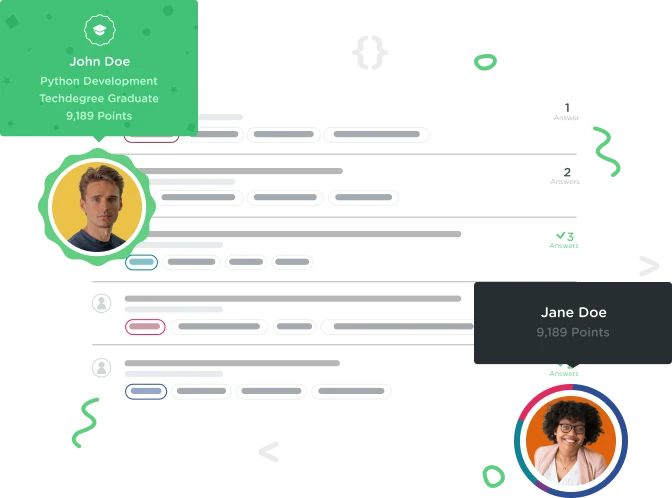
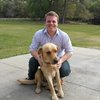
Jacob Lewis
17,355 PointsPython Collections: Lists Redux Quiz
The code challenge asks you to move the '1' to position 0. It also says you can do it in one step with .pop() and .insert(). I couldn't solve it in one step and, I did it this way:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
Your code goes below here
neo=the_list.pop(3)
new_list=the_list.insert(0, neo)
Can someone show me how to solve it in one step?
7 Answers
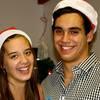
Cody Te Awa
8,820 PointsSince the .pop() method removes and also returns a value from a list, you can chuck it inside the insert statement. the insert statement is expecting a index and a value to insert at the given index. so if you were to have something like this: the_list.insert(0, the_list.pop(3)) we are popping the index 3 which is of value 1 and at the same time returning it to the insert method to therefore place it in the index 0. -Hope that helps!
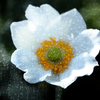
ellie adam
26,377 Pointsthe_list = ["a", 2, 3, 1, False, [1, 2, 3]]
the_list.pop(3)
the_list.insert(0, 1)
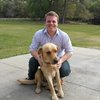
Jacob Lewis
17,355 PointsThat did help solve it. Thanks, Cody.
Charles Steinmetz
14,420 PointsYou need to pop the value by it's index number and store this in a variable. Then insert that new variable into the proper position. I did it this way:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
a_list = the_list.pop(3)
the_list.insert(0, a_list)

Tony McCabe
4,889 PointsTreehouse.com is fun, but they need to take some English course... They should of said place a new variable, then place either .pop, or .insert() The code you would place is same as Charles Steinmetz. He has the answer.
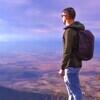
Joshua Eagle
9,778 PointsThis is old, but I was searching this issue as well and found this post. Elizabeth, your answer is close, you don't need to set the variable again. I tried running your example in python terminal and it clears out the variable "the_list" which is why it fails.
This is how you do it in one step. you wrap your pop with an insert.
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.insert(0, messy_list.pop(3))
The order of operations will evaluate what is inside of the parenthesis first i.e., messy_list.pop(3), then it will execute the insert. I hope this helps. :)
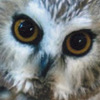
Elizabeth Henning
4,286 PointsAnother badly written question in the Python courses. I'm new to Python, and I don't know how recursion works, so I just spent fifteen very frustrating minutes trying to figure out why my answers
the_list = the_list.insert(0, the_list.pop(3))
and
new_list = the_list.insert(0, the_list.pop(3))
the_list = new_list
weren't correct.
This question should be rewritten to say something like, "Create a new variable new_list ..."
Honestly, I'm fed up with how long it's taking me to get through this track because of these poorly written challenges. The next needless frustration I encounter like this is going to send me elsewhere.