Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial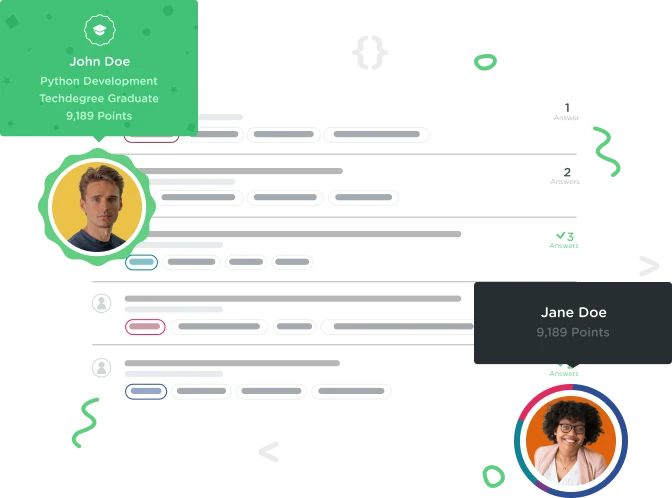
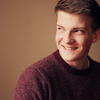
Hendrik /
13,961 PointsPython Collections - Removing Items from a list Challenge Task 2
Hi!
I'm struggling with the 2nd task and can't seem to figure out the smartest way to remove the items from the list.
I tried 2 different approaches but and the Boolean Value is the only item I can't remove.
1st way:
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.insert(0, messy_list.pop(3))
for item in messy_list:
if not isinstance(item, int):
messy_list.remove(item)
2nd way:
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.insert(0, messy_list.pop(3))
for item in messy_list:
try:
item += 1
except TypeError:
messy_list.remove(item)
The code works with the strings and lists but it doesn't remove the Boolean Value. Can somebody of you explain me why?
Thank you!
4 Answers
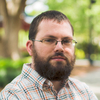
Kenneth Love
Treehouse Guest TeacherDon't be clever, just remove the value you want to remove. The smartest, and most Pythonic way, is to just do exactly what you want the first time.
That said, there is a way to do it with a loop but you'll need to use type()
instead of isinstance()
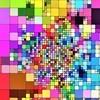
james south
Front End Web Development Techdegree Graduate 33,271 Pointsin both cases False is evaluating to 0, so isinstance says it's an int and the try block is succeeding in adding 1 to it.
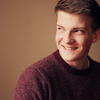
Hendrik /
13,961 PointsThank you! What do you think would be the best solution to that?
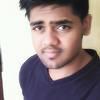
Saiteja Vemula
7,162 PointsSir, I've trouble with this code challenge. Here's my code
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
messy_list.insert(0,messy_list.pop(3))
i = 0
while i < len(messy_list) :
if not type(i) == type(messy_list[i]):
del messy_list[i]
i += 1
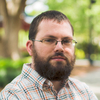
Kenneth Love
Treehouse Guest TeacherYou're changing the list as you go through it in a loop, which leads to skipped indexes. You'll need either a copy of the list or to just delete the items you want deleted instead of trying to solve this in a clever fashion.
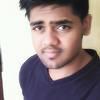
Saiteja Vemula
7,162 PointsThank you sir for notifying me that indexes were skipped when I delete a value from the list while looping. I've solved the Task like this way and it worked.
messy_list = ["a", 2, 3, 1, False, [1, 2, 3]]
messy_list.insert(0,messy_list.pop(3))
count = 0
i = 0
while i < len(messy_list) :
if not type(i) == type(messy_list[i]):
del messy_list[i]
messy_list.insert(i,'x')
count += 1
i += 1
while count:
count -= 1
messy_list.remove('x')

Jennifer Mitchell
1,831 PointsYou don't need loops or tries to solve task 2. Just use del and the index of the items you want to remove from the list. Be aware the index changes as you remove items.
Hendrik /
13,961 PointsHendrik /
13,961 PointsAlright thank you! :)