Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial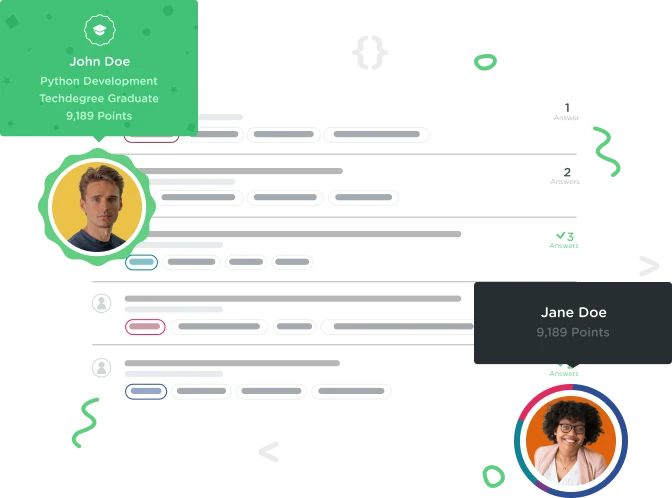

Krishna Pratap Chouhan
15,203 PointsPython Collections. Return Dictionary out of string.
Why does the following first code passes where as the second code does not.
#PASSES
def word_count(arg):
var_dict = {}
arg = arg.lower().split()
for a in arg:
if a in var_dict:
var_dict[a]+=1;
else:
var_dict[a] = 1;
return var_dict
#FAILS
def word_count(arg):
var_dict = {}
arg = arg.lower().split(' ')
#or arg = arg.lower().split(" ")
for a in arg:
if a in var_dict:
var_dict[a]+=1;
else:
var_dict[a] = 1;
return var_dict
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(arg):
var_dict = {}
arg = arg.lower().split()
for a in arg:
if a in dic:
var_dict[a]+=1;
else:
var_dict[a] = 1;
return var_dict
1 Answer
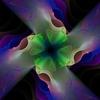
Travis Howk
7,169 PointsThis is from the python methodology:
If the separator is not specified or is None, [...] runs of consecutive whitespace are regarded as a single separator.
Both executions of your code return identical strings whenever you type in normal stuff. However, they no longer execute the same code whenever you enter a string that consists of only white space. Example:
Using the empty string "", your first algorithm will return an empty dictionary {} as desired. The second algorithm, however, returns {'':1}. Why this occurs can be found here. Basically, it just has to deal with defaults for each of the different algorithms that are launched by the different syntax in each of the respective methods.
Krishna Pratap Chouhan
15,203 PointsKrishna Pratap Chouhan
15,203 PointsThanks Travis that was really helpful. On similar lines:
why is that happening?
Travis Howk
7,169 PointsTravis Howk
7,169 Pointslen(str) = 3
This is because there are three spaces. Simple enough
len(str.split(' ')) = 4
This one is a bit more complicated. So whenever you use the split method, the string is going to be split into different bits based on where whatever you told it to split is. So what is happening here is that there aren't any characters in the string other than the spaces, and so you end up with a situation like this:
Those two above strings are equivalent. The second string shows you what the split function is doing. The reason why it has length 4 is because split(" ") splits the string " " into 4 empty strings as you can count in the above code. You can also see this for yourself if you go print str.split(" "); it will spit out an array that shows you 4 empty strings.
If you find the answers helpful, be sure to upvote them. Let me know if you have any other questions.