Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial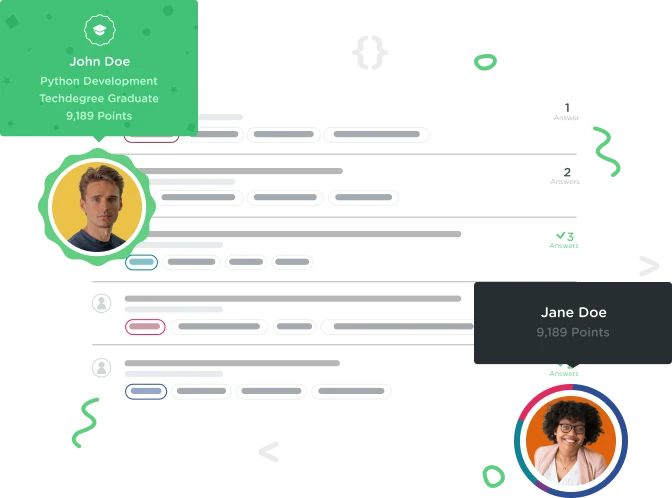

Enes artun
Courses Plus Student 2,364 PointsPython Conditional Statement Exercises
Hi. I have been doing some conditional statement exercises and I need some help with couple of them.
Write a Python program to convert temperatures to and from Celsius and Fahrenheit. [ Formula : c/5 = f-32/9 [ where c = temperature in celsius and f = temperature in fahrenheit ] Expected Output : 60°C is 140 in Fahrenheit 45°F is 7 in Celsius
temp = input("Input the temperature you like to convert? (e.g., 45F, 102C etc.) : ")
degree = int(temp[:-1])
i_convention = temp[-1]
if i_convention.upper() == "C":
result = int(round((9 * degree) / 5 + 32))
o_convention = "Fahrenheit"
elif i_convention.upper() == "F":
result = int(round((degree - 32) * 5 / 9))
o_convention = "Celsius"
else:
print("Input proper convention.")
quit()
print("The temperature in", o_convention, "is", result, "degrees.")
degree = int(temp[:-1]) i_convention = temp[-1] This part is I don't understand. How can temp be a list when it is a string?
Write a Python program that accepts a word from the user and reverses it.
word = input("Input a word to reverse: ")
for char in range(len(word) - 1, -1, -1):
print(word[char], end="")
print("\n")
for char in range(len(word) - 1, -1, -1) Start,stop and range. But len means the number of words. It should omit one letter because of that but it does not. How?
Write a Python program that takes two digits m (row) and n (column) as input and generates a two-dimensional array. The element value in the i-th row and j-th column of the array should be i*j. Note : i = 0,1.., m-1 j = 0,1, n-1.
row_num = int(input("Input number of rows: "))
col_num = int(input("Input number of columns: "))
multi_list = [[0 for col in range(col_num)] for row in range(row_num)]
for row in range(row_num):
for col in range(col_num):
multi_list[row][col]= row*col
print(multi_list)
multi_list = [[0 for col in range(col_num)] for row in range(row_num)]
I don't understand this variable at all. How does it work?
Write a Python program that accepts a sequence of comma separated 4 digit binary numbers as its input. The program will print the numbers that are divisible by 5 in a comma separated sequence. Sample Data : 0100,0011,1010,1001,1100,1001 Expected Output : 1010
items = []
num = [x for x in input().split(',')]
for p in num:
x = int(p, 2)
if not x%5:
items.append(p)
print(','.join(items))
num = [x for x in input().split(',')] for p in num: x = int(p, 2) if not x%5: items.append(p)
num list is I can't make sense of. Other part is if statement. Question ask me to find digits that can be divided by 5. It says not x%5. How does it work?
Write a Python program to print the alphabet pattern 'D'. Expected Output:(D looks little off becuase I had to type it. Looked weird when I tried to paste it.)
* * * *
* *
* *
* *
* *
* *
* * * *
result_str="";
for row in range(0,7):
for column in range(0,7):
if (column == 1 or ((row == 0 or row == 6) and (column > 1 and column < 5)) or (column == 5 and row != 0 and row != 6)):
result_str=result_str+"*"
else:
result_str=result_str+" "
result_str=result_str+"\n"
print(result_str);
There is definetly an easy way to do this with a function but I want to understand this part:
for column in range(0,7):
if (column == 1 or ((row == 0 or row == 6) and (column > 1 and column < 5)) or (column == 5 and row != 0 and row != 6)):
1 Answer
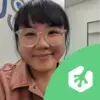
Rachel Johnson
Treehouse TeacherHey there! Some very good questions there.
Celsius > Fahrenheit question
Bracket notation, like [-1]
can be used for more than just lists. In this example, it's grabbing the last character of the temp
string, which is expected to be F or C
Reverse word question
This comes down to the fact that indexes start at 0. Let's say we use the word "chicken".
len(word)
would be 7, and so len(word)-1
would be 6
. This range would start at 6
The next -1
is the stopping point. Because range()
excludes the given value, so 0
is included in the range
The final -1
is the step, so this returns the range of 6 down to 0 (inclusive) counting backward
So the final range of numbers produced is 6, 5, 4, 3, 2, 1, 0
, which targets all 7 letters of "chicken"
Two-dimensional array question
This is a Python List comprehension. There is a new Workshop that is about to be released that goes into depth about this. In the meantime, this Workshop gives an overview of how List Comprehensions work
-
for col in range(col_num)
: This is a loop that iterates over the range of values from 0 tocol_num - 1
. The variablecol
takes on each value in this range one at a time. -
0 for col in range(col_num)
: This expression assigns the value 0 to each iteration of thecol
variable. In other words, it creates a list containingcol_num
number of 0s. -
for row in range(row_num)
: This is another loop that iterates over the range of values from 0 torow_num - 1
. The variablerow
takes on each value in this range one at a time. -
[0 for col in range(col_num)]
: This list comprehension creates a list ofcol_num
number of 0s. It's essentially the same expression as step 2. -
[[0 for col in range(col_num)] for row in range(row_num)]
: This is a nested list comprehension where the outer loop iterates over the range of values from 0 torow_num - 1
, and for each iteration, the inner loop creates a list ofcol_num
number of 0s. The result is a 2-dimensional list (a list of lists) withrow_num
rows andcol_num
columns, where each element is 0.
In summary, the line of code creates a 2D list filled with zeros, with dimensions specified by row_num
and col_num
.
Binary Numbers Question
This is also a Python List Comprehension. Be sure to refer to the Workshop mentioned above (and watch out for the new one that's coming out soon!).
This line produces a list of values. The input()
is split into a list by the ,
delimiter. Each of these (ie, 0100
, 0011
, etc) is appended to the new num
list
The %
operator is the modulo. Read more about the modulo here. x%5
will result in an integer. Positive numbers are treated as True
, while 0
is treated as False
. Since something that is divisible by 5 will return 0
, this conditional will return True
if x
is divisible by 5, because not 0 (False)
evaluates to True
'D' pattern question
-
for column in range(0, 7)
: This is a loop that iterates over the range of values from 0 to 6 (inclusive) using the variablecolumn
. -
if (column == 1 or ((row == 0 or row == 6) and (column > 1 and column < 5)) or (column == 5 and row != 0 and row != 6)):
This condition determines whether the code inside the if statement block should be executed. It combines three different conditions using logical operators (or
andand
): -
column == 1
: This checks if the value ofcolumn
is equal to 1. - The first part,
(row == 0 or row == 6)
, checks if the value ofrow
is equal to 0 or 6. -
(column == 5 and row != 0 and row != 6)
: This condition checks if the value ofcolumn
is equal to 5 and if the value ofrow
is not equal to 0 and not equal to 6. - If any of these conditions evaluate to true, the code inside the if statement block will be executed.
-
print("*")
: This line prints an asterisk character (*
). If the conditions in the if statement are true, the code inside the if statement block will be executed, and an asterisk will be printed.
To summarize, this code snippet iterates over the range of values from 0 to 6 using column
, and for each value of column
, it checks the conditions specified in the if statement. If any of the conditions are true, it prints an asterisk.
Enes artun
Courses Plus Student 2,364 PointsEnes artun
Courses Plus Student 2,364 PointsThank you. List comprehension still doesn't make much sense unfortunately even after watching the vid so I'll do some exercises about that.
Rachel Johnson
Treehouse TeacherRachel Johnson
Treehouse TeacherHey Enes artun !
The new Python Comprehensions course has been released yesterday! Be sure to check it out to have a solid understanding of List Comprehensions!
https://teamtreehouse.com/library/python-comprehensions-3