Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial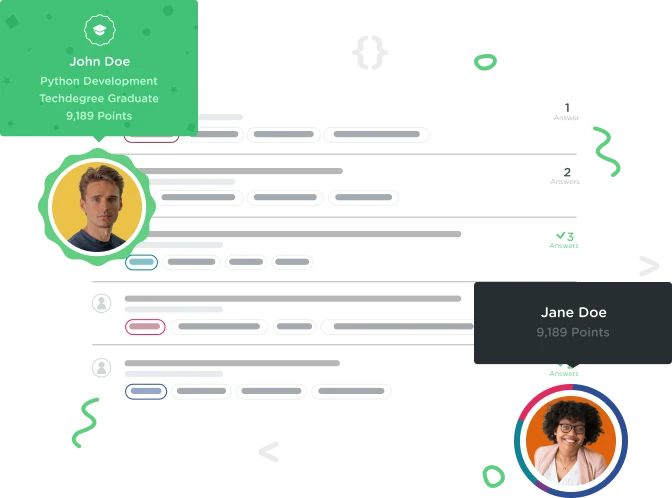
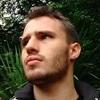
Byron Farrell
5,700 PointsPython Constructors
Hi,
I am following a tutorial for Django and I am a bit confused about some of the code
BELOW IS CODE IN TUTORIAL
q = Question(question_text="What's new?", pub_date=timezone.now())
MY MODELS CLASS
from future import unicode_literals
from django.db import models
class Question(models.Model): question_text = models.CharField(max_length=200) pub_date = models.DateTimeField('date publushed')
class Choice(models.Model): question = models.ForeignKey(Question, on_delete=models.CASCADE) choice_text = models.CharField(max_length=200) votes = models.IntegerField(default=0)
MY QUESTION
In my models module the class Question inherits from the class Model. there is no constructor for this class but in the code above mine you can create an object and add keyword arguments when instantiating a object ( q = Question(question_text="What's new?", pub_date=timezone.now()) ). My question is how does this work because I have not defined a constructor?? but it still seems to work.
1 Answer

matth89
17,826 PointsByron,
When you inherit from models.Model, you are inheriting the init method defined on Model in the Django source code, which allows you to set keyword arguments when instantiating your class. This is one of the many ways in which Django keeps you from having to re-invent the wheel while building your models.
I hope this helps!
Byron Farrell
5,700 PointsByron Farrell
5,700 PointsThanks for replying Matt,
I created a class A and a class B(A). class A had a constructor with name="no name" and class B had no constructor. When I tried to create a object i.e. obj = B(name = "Jim") I got an error because class B didn't have a defined constructor. Why does this not work in python but it works fine with the django class Model?
Is there a way I can make a sub class automatically inherit the constructor from the base class without defining a constructor like the django Model class does?
Hope this makes sense...I am still very new to python and django
matth89
17,826 Pointsmatth89
17,826 PointsSubclasses will inherit the init method from the base class in python. Could you post your code so I can help you troubleshoot?
Byron Farrell
5,700 PointsByron Farrell
5,700 Pointsclass A: def init(self, **kwargs): for key, value in kwargs.items(): setattr(self, key, value)
class B(A): pass
objA = A(name = "Bob") print(objA.name)
objB = B(name = "Jim") print(objB.name)
I checked this code and it worked. I thought it wouldn't because I didn't define a def __init() in class B. Do sub classes automatically inherit its base class constructor if you don't define one in the sub class?
matth89
17,826 Pointsmatth89
17,826 PointsYes they do! When you inherit from a class, you're getting everything that was defined on the original class.
Byron Farrell
5,700 PointsByron Farrell
5,700 PointsAhh, thanks for clearing that up for me Matt, I come from a java background where you have to define a constructor then call the super constructor so the python way of doing it confused me a bit
matth89
17,826 Pointsmatth89
17,826 PointsGlad to help!
Just to be clear though, Python does have a super as well. You would use it if you were overriding init on your subclass, but still wanted to get everything from the base class init. You'll find yourself doing that in Django quite a bit. More on that here:
https://www.pythonforbeginners.com/super/working-python-super-function