Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial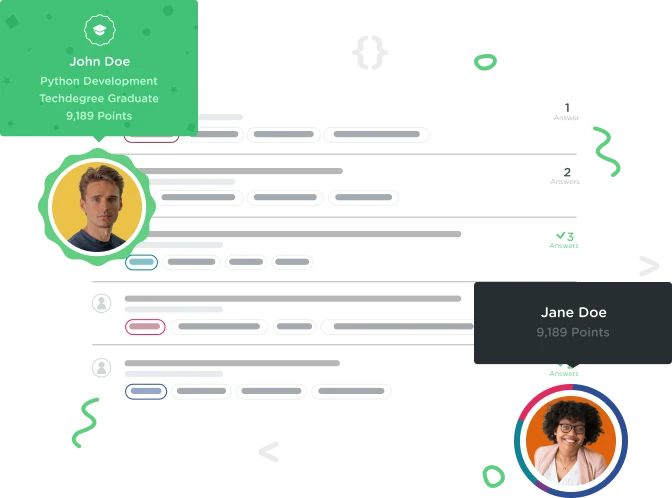

Jim Sweeney
3,050 PointsPython counting help
I need to create 2 new variables, one named estimate that holds the rounded number of decades you've lived and, one named summary that adds the string version of estimate into "I am {} days old! That's about {} decades!" with the correct values added to it. Here's my code.
age = int(38)
days = age * 52 * 7
decades = age / 10
estimate = round(decades)
summary = ("I am {} days old! That's about {} decades!", (days, str[estimate]))
I keep getting an error message saying that Task one isn't passing. It passed with the first three steps, but not the final one. Any help would be greatly appreciated. Thanks.
4 Answers
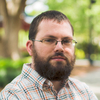
Kenneth Love
Treehouse Guest TeacherYour first solution is fine with the exception of your last line. Since it contains some invalid code, the code challenge runner thinks the first task is failing. Let's look at that line. (you don't need to cast 38 to an int in the first line, either, but that won't make anything fail)
summary = ("I am {} days old! That's about {} decades!", (days, str[estimate]))
OK, so, first of all, you don't need those parentheses around everything. They won't make anything fail in this instance, but they're unnecessary and using them in other cases might cause things to fail. Let's avoid that.
summary = "I am {} days old! That's about {} decades!", (days, str[estimate])
So what's wrong now? Well, you're not calling the .format()
method at the end. Putting that comma in there creates a tuple (You'll learn about them in Python Collections) and we don't want that.
summary = "I am {} days old! That's about {} decades!".format(days, str[estimate])
This still isn't passing but it's super-close. If you run this, you'll get an error about "type is not sub-scriptable" that's about as obvious as possible. What it means is that you're trying to get something out of a base type and Python can't do that. Right now you're probably thinking "wat"? Look at the end. You did str[estimate]
which says "give me the 'estimate' index position of str
". str
is the built-in type that makes a string. And estimate
is an int. But types don't have index positions so Python blows up.
If we wanted to make estimate
into a string, we'd want to use parentheses, not brackets. str(estimate)
not str[estimate]
. But, thankfully, we don't need either of those. .format()
will turn things into strings for us.
summary = "I am {} days old! That's about {} decades!".format(days, estimate)
That ^ should pass just fine for you.

Jim Sweeney
3,050 PointsThank you for your response. I'm just starting out with python. I enter the first variable into the quiz challenge as age = 38 but it came back telling me that I need to specify that it was an integer. That's why I changed it to ago = int(38). I didn't know about the division of 2 integers and specifying the decimal point. Finally, I feel like a moron, because I forgot to add the .format and my syntax was off. Thanks for the help.
Jim
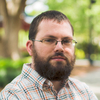
Kenneth Love
Treehouse Guest TeacherDon't feel like a moron! We all start off somewhere.
Can you make the first step tell you that age = 38
gives you a non-integer again? I haven't been able to replicate that.

Jim Sweeney
3,050 PointsI just tried the following code, incorporating your suggestions and it's still saying that it doesn't pass Task one.
age = int(38)
days = age * 52 * 7
decades = age / 10.
estimate = round(decades)
summary = "I am {0} days old! That's about {1} decades!".format(days, str[estimate])
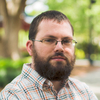
Kenneth Love
Treehouse Guest TeacherUpdated your posts for syntax highlighting
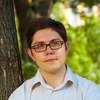
Artem Prytkov
11,932 Pointsi have a lot of questions for this code )))
1) You dont have to explicitly convert to int. If you enter number without decimal point, it
ll recognized as integer
age = 38
2) In python 2 if you divide two integers you got an integer, if you want decmal - divide by 10.0 For example
38 / 10 = 3
38 / 10. = 3.8
round(3) = 3
round(3.8) = 4
3)You don`t have to use that bunch of brackets )))
"I am {0} days old! That's about {1} decades!".format(days, str[estimate])
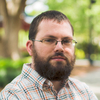
Kenneth Love
Treehouse Guest TeacherWe don't use Python 2, though, so dividing with integers gives you a float.