Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial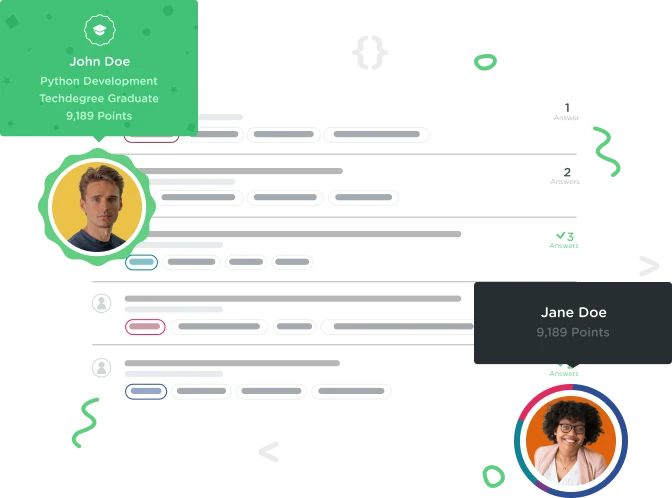
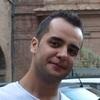
reza sajadian
718 PointsPython dictionaries
Here is my quiz,
Create a function named string_factory that accepts a list of dictionaries and a string. Return a new list build by using .format() on the string, filled in by each of the dictionaries in the list.
and here is my answer.
dicts = [ {'name': 'Michelangelo', 'food': 'PIZZA'}, {'name': 'Garfield', 'food': 'lasanga'}, {'name': 'Walter', 'food': 'pancakes'}, {'name': 'Galactus', 'food': 'worlds'} ]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(): for items in dicts: new_list = string.format(**dicts) return(new_list)
can anybody help me finding the problem? Thanks.
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory():
for items in dicts:
new_list = string.format(**dicts)
return(new_list)
2 Answers
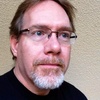
Chris Freeman
Treehouse Moderator 68,423 PointsThere were a few items to fix in your code:
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, string): # <-- add string parameter
new_list = [] # <-- initialize new_list
for items in dicts:
new_list.append(string.format(**items)) # <-- append built string using the "items" dict
return new_list # <-- indent 'return' to be inside of function definition
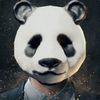
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHi there Reza. I have taken a look at your code and noticed that no arguments are required to be passed in by your function. The string_factory
function takes 2 arguments; a list of dictionaries and a string. Here is my solution to the challenge:
def string_factory(dicts, string):
new_list = []
for i in dicts: #for every dictionary in dicts
new_list.append(string.format(**i)) #format the key and value into string and append it to new_list
return new_list
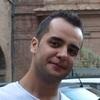
reza sajadian
718 PointsThank you Haider. It was completely helpful. Best regards.
reza sajadian
718 Pointsreza sajadian
718 PointsThank you so much Chris. It was totally helpful, and your comments are really guiding me through. I had the Append, and the function arguments missing. Best regards.
Stefan Vaziri
17,453 PointsStefan Vaziri
17,453 PointsWhy did you use the append function? Wouldn't format swap the respective words in the right place in the sentence? Thanks Chris!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsI used the
append
because the return value is expected to be alist
and it's the easiest way to add an item to the end of a list.You are correct that using
**items
has the potential of producing the key-value pairs in an arbitrary order. This is resolved by using named fields in the format string.{name}
and{food}
will receive the correct value based on the key. When using named fields order of theformat
arguments doesn't matter:Haider Ali
Python Development Techdegree Graduate 24,728 PointsHaider Ali
Python Development Techdegree Graduate 24,728 PointsI think you deserved best on this one ;).
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThanks Haider!