Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial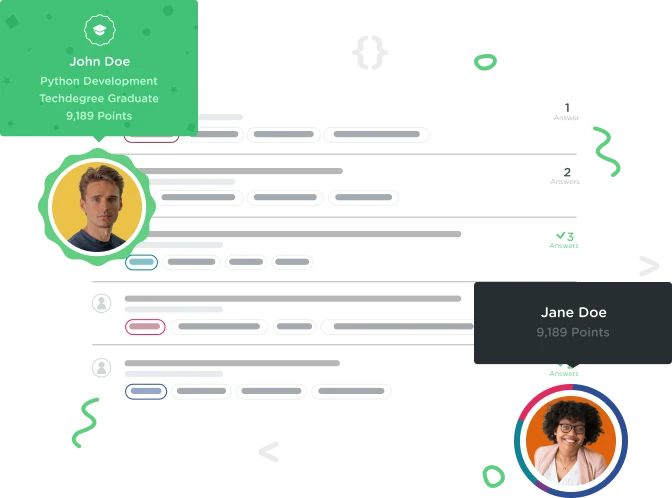

Dustin Baker
1,373 PointsPython Dictionaries / Keys
Hi Kenneth. I don't know why the following code won't work. I have tried it a bunch of different ways which I would think would all pass.
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
def members (my_dict):
my_dict = {
'LA' : 'Dodgers',
'SF' : 'Giants'
}
counts = []
for key in my_dict:
counts.append(len(my_dict[key]))
return counts
I don't see any typos...
Edit: Also the whitespace is fine in the text box, but it all runs together after I save it.
7 Answers
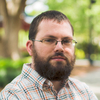
Kenneth Love
Treehouse Guest TeacherWell, you're overriding whatever's passed in for my_dict
by redefining it inside the function. You're also returning after the first time through the for
loop instead of after the for
loop is finished, so you'll only ever get one result.
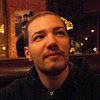
ilias Georgakopoulos
22,866 PointsI also stuck at this challenge. I tried something else that works all right but i suppose is not the way that the challenge wants to solve it.
def members(**my_dict):
return len(my_dict)
I tested that i give
members(a=1, b=2)
i will get back the number of the keys
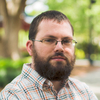
Kenneth Love
Treehouse Guest TeacherThis challenge doesn't require unpacking the dictionary at all. Some of the keys in the list will not be in the dict, so you have to check for membership, which you can do with if key in dict:
-style code.
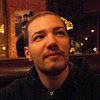
ilias Georgakopoulos
22,866 PointsI still can't figure out the solution :( So far i am sure that i have to use 2x arguments as you mentioned. One for the dictionary and one for the list of keys.
def members(my_dict, my_keys):
Then i have to check how many of my_keys are in my_dict with if my_key in my_dict:
counts = []
if my_key in my_dict:
counts.append(len(my_dict[my_key]))
return counts
I know that is not right as it is. Please someone let me know where i am right/wrong and what should i try. Thanks in advance.
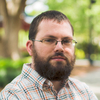
Kenneth Love
Treehouse Guest TeacherYou are so close. You don't want a list of key lengths, you want an integer that counts the number of keys. So if three of the keys are in the dict, the count would be three.
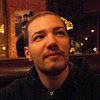
ilias Georgakopoulos
22,866 PointsFirst i want to thank you for your fast response.
I changed my code
def members(my_dict, my_key):
counts = 0
for my_key in my_dict:
counts += 1
return counts
It says Bummer! Expected 2, got 6.
Damn it i know that i am close.
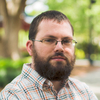
Kenneth Love
Treehouse Guest TeacherYou are super close. Only increment the count if the key is in the dict.
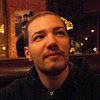
ilias Georgakopoulos
22,866 PointsI finally did it.
def members (my_dict, my_key):
counts = 0
for item in my_key:
if item in my_dict.keys():
counts += 1
return counts

Andy Manning
628 PointsHey, obviously I can see why that is right above but I'm puzzled as to why this is not (returns 0)
def members(my_dict, my_key):
count = 0
for item in my_key:
if item in my_key == item in my_dict:
count += 1
return count
Thanks!
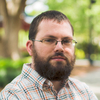
Kenneth Love
Treehouse Guest Teacherdef members(my_dict, my_key):
count = 0
for item in my_dict: # item is a key in the dict
if item in my_dict == item in my_key: # This *looks* the same as: if (item in my_dict) == (item in my_key) but actually isn't
count += 1
return count
Your comparison is going to return False
because Python isn't reading it the way you're thinking of it. If you put in the parentheses from my comment, it'll probably work but this totally isn't the right, Pythonic way of solving the problem. You're close, though.
You don't need to do the in
comparison twice.

Andy Manning
628 PointsThanks Kenneth for the super quick answer. I get it now... seems obvious when you know!