Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial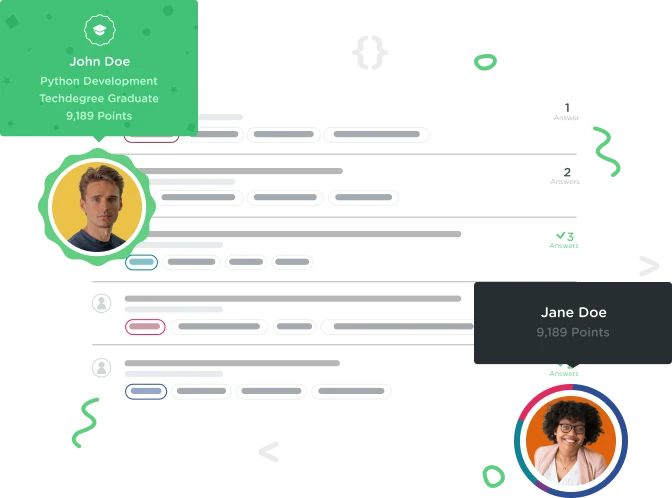
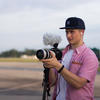
Christopher Johnson
12,829 PointsPython For Loop
I understand everything about how a for loop works except in this example...
state_names = ['Alabama', 'California', 'Oklahoma', 'Florida']
vowels = list('aeiou')
output = []
for state in state_names:
state_list = list(state.lower())
In the for statement, I don't understand where state is coming from... I see the obvious that state_names is present, but what exactly is 'state'? It seems like python knows that state is tied to the strings within state_names.
2 Answers
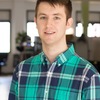
Ethan Lowry
Courses Plus Student 7,323 PointsJust think of it as a convenient shortcut to prevent you having to manually assign the current item in the loop to a variable yourself. In this example, 'state' could be called anything, it doesn't matter.
So at any time in the loop, you can refer to state
in order to access the current item of the array being looped over.
Hope that clears it up, these kind of for loops tend to confuse beginners but they are very intuitive and super handy once you get used to them.
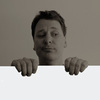
Sean T. Unwin
28,690 PointsGood description, Ethan Lowry . +1
In the hopes to simplify further, in case anyone is still having trouble understanding, let's go over the key points.
The for
loop receives an object which has items in it. Then the loop does something to each item consecutively (one at a time). In many cases,the loop considers all items in the object and starts at the beginning (the first item) of the object and goes to the end (the last item).
To illustrate, let's say:
- There is a car wash; it washes each car one after another
- Therefore, the car wash consists of a loop
- The Object is the line of cars or set of customers, which is to say all the cars in the line waiting to enter the car wash
- The car that enters the car wash is the current priority of the car wash
When a car enters the car wash some things happen. This is what happens inside the for
loop. When a car is inside the car wash that car (the current item or index) gets washed. When that is done, the car exits and the next car in the line up enters. This process repeats until each item has been dealt with.
So in programming terms we can say,
- In the context of a car wash:
-
car_wash
consider the line of customers waiting to get their car washed - when a car enters the car wash then wash the car
- we will call this car the
current_car
. This is arbitrary, we can can name it whatever we like but it makes sense to keep within the context of the object it is inside of
- we will call this car the
- when the car is washed it exits and the next one enters
-
- Repeat steps 2 and 3 until there are no more cars in the line-up
In Python we can say:
1 line_of_cars_to_be_washed = ['car1', 'car2', 'car3', 'car4', 'car5']
2 # within the context of car_wash
3 # wash each car in the line
4 for current_car in line_of_cars_to_be_washed:
5 # the current car enters the car wash
6 car_wash.wash_car(current_car)
7 # car is clean and leaves
8 # if there are still customers waiting to get car washed
9 # then go back to line 4 until all cars are washed
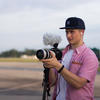
Christopher Johnson
12,829 PointsLowry's explanation was good, but I do appreciate how you broke down the for loop. I understand how it works, but I find it a good idea to learn multiple explanations to further understand it a bit more, so thank you!
Christopher Johnson
12,829 PointsChristopher Johnson
12,829 PointsHey Thanks! I am use to Javascript, so this was a bit different for me. That makes total sense and adds a lot of ease for programming! Great description!