Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial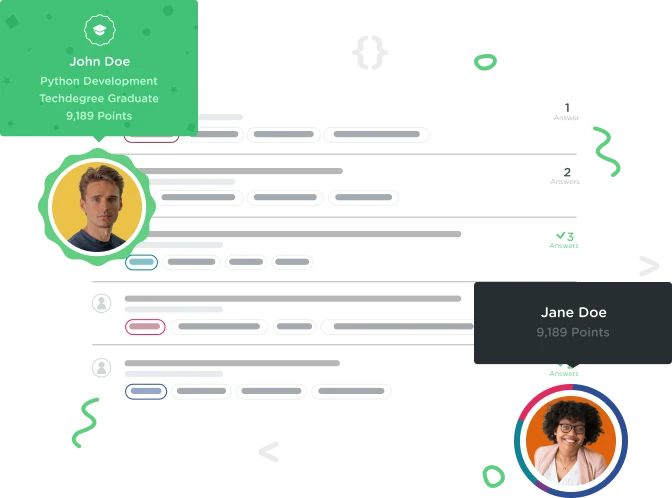
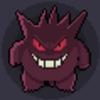
Iris Avalon
14,477 Pointspython game.py doesn't run
So, I've been staring at my code for a while, and I just can't see what the issue is here. The python interpreter isn't spitting any errors either..
import sys
from player import Player
from monster import Dragon
from monster import Goblin
from monster import Troll
class Game:
def setup(self):
self.player = Player()
self.monsters = [
Goblin(),
Troll(),
Dragon()
]
self.monster = self.get_next_monster()
def get_next_monster(self):
try:
return self.monster.pop(0)
except IndexError:
return None
def hit(self, creature):
if creature == 'monster':
self.monster.hit_points -= 1
elif creature == 'player':
self.player.hit_points -= 1
def monster_turn(self):
if self.monster.attack():
print('{} is attacking!'.format(self.monster))
if input('Dodge? Y/N ').lower() == 'y':
if self.player.dodge():
print('{} dodged the attack!'.format(self.player))
else:
print('{} got hit anyway'.format(self.player))
self.hit('player')
else:
print('{} hit you for 1 point!'.format(self.monster))
self.player_hit('player')
else:
print('{} isn\'t attacking this turn'.format(self.monster))
def player_turn(self):
player_choice = input("[A]ttack, [R]est, [Q]uit? ").lower()
if player_choice == 'a':
print("You're attacking {}: ".format(self.monster))
if self.player.attack():
if self.monster.dodge():
print('{} dodged your attack!'.format(self.monster))
else:
if self.player.leveled_up():
self.hit('monster')
else:
self.hit('monster')
print('You hit {} with your {}: '.format(
self.monster, self.player.weapon))
else:
print('You missed!')
elif player_choice == 'r':
print('You rest and gain 1 hit point!')
self.player.rest()
elif player_choice == 'q':
sys.exit()
else:
self.player_turn()
def cleanup(self):
if self.monster.hit_points <= 0:
self.player.experience += self.monstre.experience
print('You killed {}: '.format(self.monster))
self.monster = self.get_next_monster()
def __init__(self):
self.setup()
while self.player.hit_points and (self.monster or self.monsters):
print('\n'+'='*20)
print(self.player)
self.monster_turn()
print('-'*20)
self.player_turn()
self.cleanup()
print('\n'+'='*20)
if self.player_hit_points:
print('You win!')
elif self.monster or self.monsters:
print('You lose')
sys.exit()
Game()
When I run python game.py , the interpret does nothing (just starts a new line in terminal. No python error at all. It just seems to end immediately.
I've probably got a painfully simple logic error in here somewhere, but I can't find it.. any help? :D
3 Answers
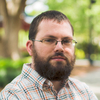
Kenneth Love
Treehouse Guest Teachercleanup()
and __init__()
seem to be indented too far and are inside of player_turn()
. This makes them uncallable for how we've written everything. Bring them back out one level of indentation?
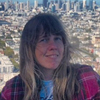
Cissie Scurlock
11,072 PointsI can't see why your game won't run but I did notice a typo in your clean up function:
self.player.experience += self.monstre.experience

William Higgins
5,904 Pointsdef get_next_monster(self): try: # this should be monsters, not monster return self.monster.pop(0) except IndexError: return None