Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial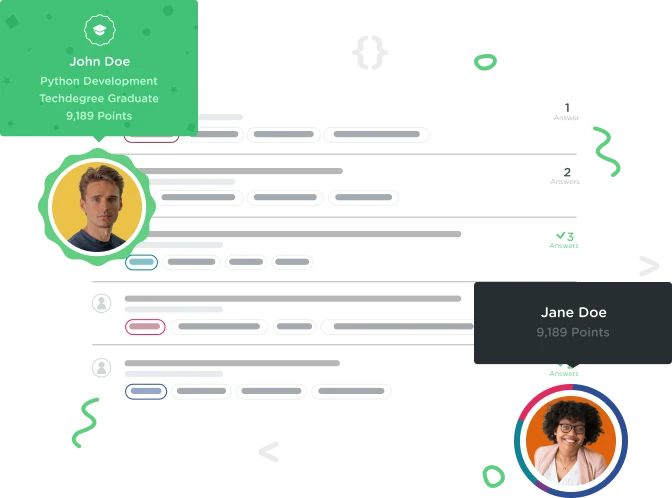

Peter Evemy
1,002 PointsPython: How can you print strings one character at a time?
Hey,
I've been practising python by writing a little text based adventure game. I'm trying to find a way to print out the dialogue in the game one character at a time, kind of like the old pokemon gameboy games.
I've looked around but can't find anything that works. I guess I have to define a function for the print that uses time, which has a sleep(0.02) between each character. Maybe using a loop to pick out each len() in the text and print them? Or convert the string into a list and print it that way?
Very new to python so all help appreciated.
Thanks a lot!
2 Answers
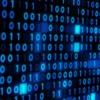
Alexander Davison
65,469 PointsGrigorij Schleifer's solution works, but it's easier to simply use the end
parameter on print
:
import time
for x in "my string":
print(x, end='')
time.sleep(0.05)
By default, end is a newline, therefore Python by default prints a newline after the string, but you can easily change it to have no ending.

Peter Evemy
1,002 PointsThank you a lot for this :)!
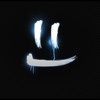
Grigorij Schleifer
10,365 PointsHi Peter, I am not sure I understand your question but here is a very basic code that prints a string one character after another.
import time
import sys
string = "Some sting"
for char in string:
# these two lines prevent python from including a new line after every character
sys.stdout.write(char)
sys.stdout.flush()
time.sleep(0.500)
Let me know what you think ...

Peter Evemy
1,002 PointsAmazing! This was exactly what I was looking for! Thanks so much!
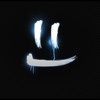
Grigorij Schleifer
10,365 PointsHi Peter, you have been asking for a method that does the same thing.
import time
import sys
word = "Some sting"
def character_timer(string):
for char in string:
sys.stdout.write(char)
sys.stdout.flush()
time.sleep(0.500)
character_timer(word)
I hope that helps
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsVery nice. I am glad I could help. Good luck with your program. If you like suggestions and comments, feel free to post your program here in the forum.