Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial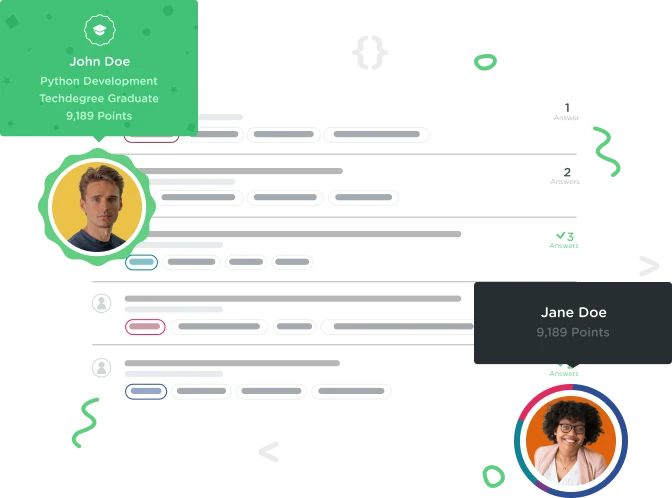

Michael Cook
5,864 Points(Python) How to print from lists using for loop based on the first letter of each item.
Hi, so I just got done watching the video and I'm doing this problem where I have to get it to print only continents starting with the letter A, and I have no clue on how to attack this.
I started by trying to replace 'continent' in 'for continent in continents:' with a "A" but that just returned a SyntaxError , I also heard you can get the first letter of an item in the list with it (for example continents[0][0]), but all the methods I've tried just never worked.
I rewatched the video before this several times and it never really became clear to me on how to solve this coding challenge. It showed how you can get the first letter in the string by doing string_name[0], but I'm not sure how that will work in lists. my_list[0][0] will display the first letter in first string in the list, but I'm not sure how to use that in order to solve this problem.
I don't really even understand how these 'lists' work, so sorry if I'm missing anything obvious.
Thanks for the help.
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
for continent in continents:
print("* " + continent)
2 Answers

ovtwvgmbwl
5,440 PointsHi Michael!
You're going to want to introduce flow control to answer this problem. Your task here is:
"only print continents that begin with the letter "A"."
The best way to tackle this problem is to check each continent string in the list and see if the first value is equivalent to the character A. You can iterate over strings similar to how you'd iterate over lists, and you can access index values of strings in much the same way. For example with the string "Hello, World" you can access the character "W" with an index of 7. I've included a possible solution to the challenge below. Let me know if you have more questions.
continents = [
'Asia',
'South America',
'North America',
'Africa',
'Europe',
'Antarctica',
'Australia',
]
#1. Iterate over the list of continents using a FOR loop
for continent in continents:
#2. For each iteration, check if the first character of that iteration (at index 0) is equivalent to "A"
if continent[0] == "A":
#3. If the first character is equivalent to "A", print out the continent name as a whole.
print("*", continent)

Michael Cook
5,864 PointsWoah! That reply was lightning quick, that also makes so much sense, thanks a lot! I thought about replacing the for statement with an if statement, but I didn't think about combining the two, it really does just click all together.
Thanks again!