Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial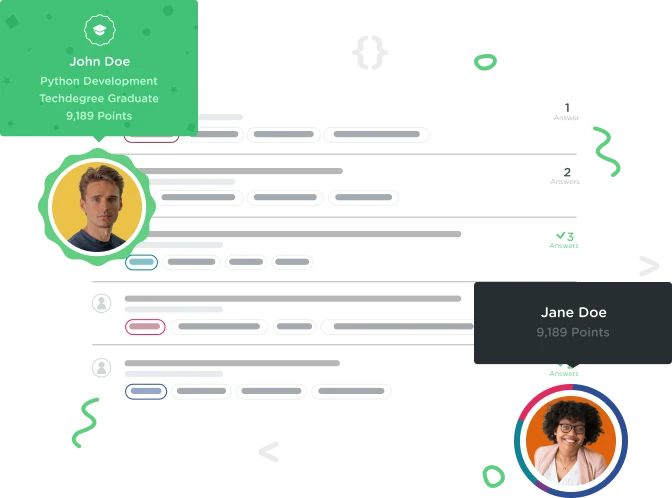

Larissa Banitt
681 PointsPython Iterable
I have a question that goes "Make a function named first_4 that accepts an iterable as an argument and returns the first 4 items in the iterable." and am stuck on what to do. How do I make the iterable?
4 Answers
Nyasha Chawanda
7,946 Pointsyou can try this
def man(iterable): return iterable[:4]
this will take an argument which is iterable and return the first four values of that iterable
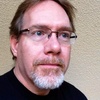
Chris Freeman
Treehouse Moderator 68,423 PointsIn iterable is anything that can be iterated upon. Such as a list, str, tuple. See docs: iterable
If you just want the first for items you can use the indexing [:4]
, such as:
In [8]: tup = (1, 2, 3, 4, 5, 6)
In [9]: tup[:4]
Out[9]: (1, 2, 3, 4)
If you want to use a function:
In [10]: def first_4(iterable):
....: return iterable[:4]
....:
In [11]: first_4(tup)
Out[11]: (1, 2, 3, 4)
You could also create a for-loop and inspect each item if you need operate on each item individually:
In [15]: def first_4(iterable):
for item in range(4):
# operate on item
print(iterable[item])
....:
In [16]: first_4(tup)
1
2
3
4
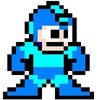
Robert Richey
Courses Plus Student 16,352 PointsHi Larissa,
That's describing what kind of data will be passed to your function. So, your function will receive an iterable object, which means you can put it in a loop and look at each element.
my_list = [1, 2, 3] # this is an iterable
# function with a silly name that accepts one argument - which I am choosing to call itr, for iterable
def foo(itr):
for element in itr:
# lets see what's inside itr!
print(element)
Hope this helps!
Cheers

Larissa Banitt
681 PointsThis is what I entered, but it isn't working: first_4 = (1, 2, 3, 4, 5)
first_4 [:4]
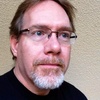
Chris Freeman
Treehouse Moderator 68,423 PointsThere should not be a space in between: first_4[:4]
, but the interpreter is flexible:
first_4 = (1,2,3,4,5)
In [18]: first_4 [:4]
Out[18]: (1, 2, 3, 4)
In [19]: first_4[:4]
Out[19]: (1, 2, 3, 4)

Larissa Banitt
681 PointsIt still says it's incorrect, but it just says try again, so I don't know how I should change it
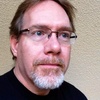
Chris Freeman
Treehouse Moderator 68,423 PointsCan you post what you have entered and the error message given back. Sometimes it's the line above that causes the error.
Larissa Banitt
681 PointsLarissa Banitt
681 PointsThank worked, thank you!