Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial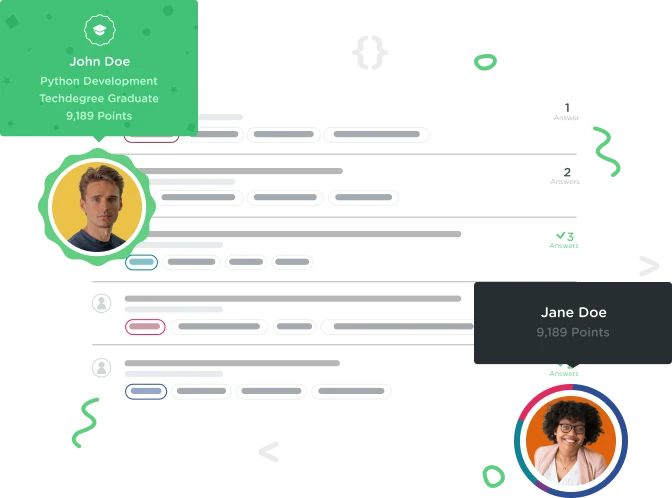

Jorge Dominguez
12,632 PointsPython join() function
I cant find any solution
shop_list = [1,2,3]
def add_list(item):
shop_list.append(item)
print('+'.join(shop_list))
What should I do?
1 Answer

Joseph Kato
35,340 PointsHi Jorge,
There's essentially three parts to this challenge:
Creating a function which takes a list as an argument.
Summing the elements of the passed-in list.
Returning the calculated sum.
You've got the first step pretty much done. For the second step, you want something along the lines of this:
list_sum = 0
for item in shop_list:
list_sum = list_sum + item
This iterates through the list, adding each element to the variable sum. Finally, you want to return the calculated sum with:
return list_sum
Putting it all together, we have:
def add_list(shop_list):
list_sum = 0
for item in shop_list:
list_sum = list_sum + item
return list_sum
There's another option, too. Python has a built-in sum
function which could help us turn the above into:
def add_list(shop_list):
return sum(shop_list)