Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial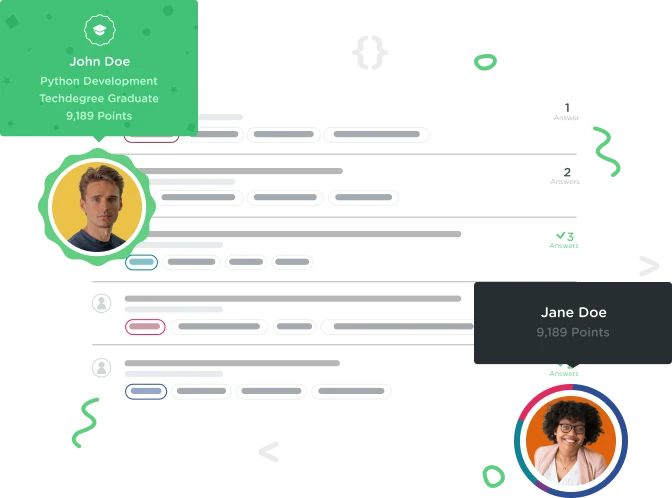

thomas2017
1,342 PointsPython Letter Game Import and split words into list
Hello, I'm trying to import a list from another file to feed it into my letter game. This is how it appears in the note file apple, banana, orange
I tried several ways but it just doesn't work.
1st method gives me an error 'list' object has no attribute 'split'.
2nd method gives me: [['apple', ' banana', ' orange', '']]
-I don't know if this is a list within a list or what, does that mean words was already a
list and I don't need to make it into a list?
3rd method: ['apple', 'banana', 'orange,']
-I don't know why there is a comma at the end of orange
4th method: apple, banana, orange,
['a', 'p', 'p', 'l', 'e', ',', ' ', 'b', 'a', 'n', 'a', 'n', 'a', ',', ' ', 'o', 'r', 'a', 'n', 'g', 'e', ','] -on the first line I guess this is a string, but I thought I converted it to a list
5th method: ['apple', ' banana', ' orange', '']
I don't know why there is extra space before the word, and why there is a quote after orange
import os
import random
import sys
import csv
#1st method gives me an error 'list' object has no attribute 'split'.
file = open('letter_game_list.txt', 'r')
k = (file.readlines())
k.split(", ")
print(list(k))
#2nd method gives me: [['apple', ' banana', ' orange', '']]
words = [word.split(',') for word in open("letter_game_list.txt", "r").readlines()]
print(list(words))
#3rd method: ['apple', 'banana', 'orange,']
#I guess this is a string, but I thought I used list for word
with open('letter_game_list.txt', 'r') as f:
for line in f:
words = list(line.split(', '))
print(words)
f.close()
#4th method: apple, banana, orange,
#['a', 'p', 'p', 'l', 'e', ',', ' ', 'b', 'a', 'n', 'a', 'n', 'a', ',', ' ', 'o', 'r', 'a', 'n', 'g', 'e', ',']
def get_file(words):
try:
file = open('letter_game_list.txt')
except IOError:
print("Unable to get the list from the file letter_game_list.txt")
sys.exit()
else:
x = file.read()
file.close()
print(x)
#5th method: ['apple', ' banana', ' orange', '']
with open('letter_game_list.txt', 'r') as csvfile:
letter_list = csv.reader(csvfile)
for row in letter_list:
print(row)
2 Answers
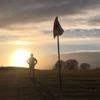
Stuart Wright
41,120 PointsI think something like this would work:
with open('letter_game_list.txt', 'r') as input_file:
words = input_file.read().split(', ') # The split method creates a list so no need to do anything else
# If you want to print them out
for word in words:
print(word)
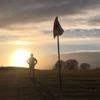
Stuart Wright
41,120 PointsI added an extra line which would handle this:
with open('letter_game_list.txt', 'r') as input_file:
words = input_file.read().split(', ')
words = [word.strip() for word in words]
# If you want to print them out
for word in words:
print(word)
The .strip() method removes all leading and trailing whitespace from a string.
For more complicated string pattern matching, you might need to start looking into regular expressions (there's a course on Python regular expressions here on Treehouse).
thomas2017
1,342 Pointsthomas2017
1,342 PointsThanks Stuart! Ohh I was wondering what if my list of words is not neatly arranged in in commas? Like what if there is accidently 2 spaces in between some 2 words. Now the spacing is all messed up. ex. apple,_orange, _ _banana
*Now it a split.(, ) would result in ['apple', 'orange', '_banana'] I used underscores to demonstrate blank spaces. Is there way to do splits that will pick up different types of situations? Where as sometimes there are multiple spaces, or that there is something else besides commas in between 2 words?