Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial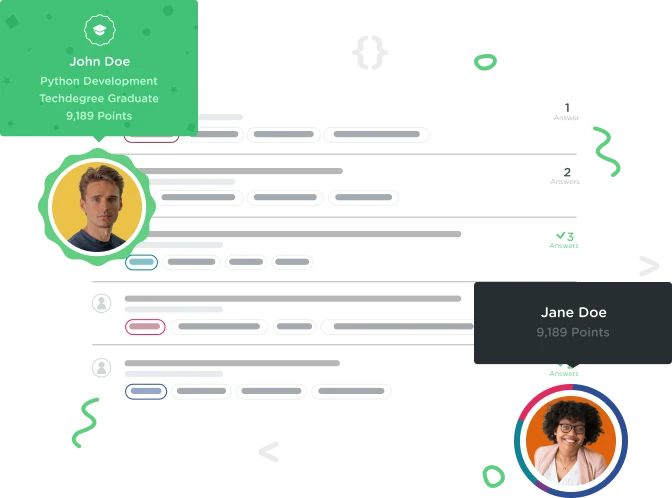

Ammar Fatihallah
7,417 Pointspython list problem
i have been stuck on this question for days. any suggestions would be greatly appreciated.
def first_4(x):
return x[0:4]
def odds(a):
return a[1::2]
def first_and_last_4(s):
return s[4:0:1]
return s[4:0:-1]
2 Answers

Ryan S
27,276 PointsHi Ammar,
Although you can have multiple return statements in a function, they need to be routed through different conditions such that you will only ever return one thing at a time. If you set up 2 consecutive return statements like you have, only the first one will actually trigger.
But to solve the 3rd part of the challenge, you can actually reuse the code you wrote in task 1. This will produce the first 4 items in the list. (You can either rewrite the code in first_4()
or you can just call the function instead of writing the same code twice. It doesn't really matter in this case since the code is so minimal, but it's always good practice to not repeat code you've already written if you can help it.).
To get the last 4, you need to start at the opposite end of the list using a negative index reference, so -4 would be the starting point. Then you will just increment normally to the end.
And finally, to add lists together you can simply use the plus sign.
def first_4(x):
return x[0:4]
def odds(a):
return a[1::2]
def first_and_last_4(s):
return s[0:4] + s[-4:]
# Alternatively
def first_and_last_4(s):
return first_4(s) + s[-4:]
Hope this helps.
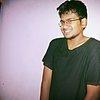
Vidhya Sagar
1,568 PointsIt should actually return it as a single list .
def first_4(arg1):
return arg1[0:4]
def odds(arg1):
return arg1[1::2]
def first_and_last_4(arg1):
first_4=arg1[0:4]
last_4_inverted=arg1[-1:-5:-1]
last_4=last_4_inverted[::-1]
return first_4+last_4