Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial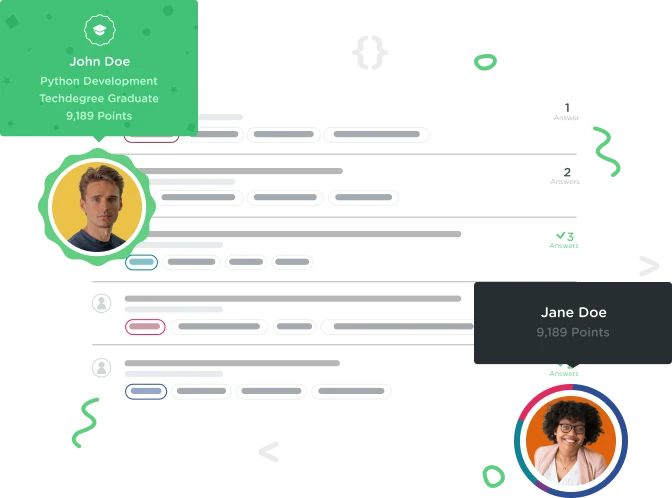

Andrew Mlamba
15,642 PointsPython Recursion
I'm trying to write a recursive function but got stuck. A really long explanation would help.
times is the number of times to repeat and data is the number or string to be repeated e.g replicate_recur(3, 5) should return [5,5,5].
Here is my attempt.
def replicate_recur(times, data):
"""This is a recursive function
that returns an array containing repetitions of the data argument"""
if not isinstance(times, int):
raise ValueError('Invalid argument')
elif not isinstance(data, (int, str)):
raise ValueError('Invalid argument')
elif times <= 0:
return []
else:
arr = []
if len(arr) == times: # base case
return arr # no recursive call
else:
return [(str(data) + ',') * times]
1 Answer
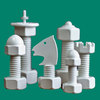
Steven Parker
230,274 PointsRemember that a recursive function will call itself, but with one or more arguments modified. For example, a function to add all numbers from 1 to a specific end value might look like this:
def add_numbers(val):
if val > 1:
return val + add_numbers(val - 1)
return 1
You should find that your function will require much less code once you structure it to just do a part of the task, and use itself to do the rest.