Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial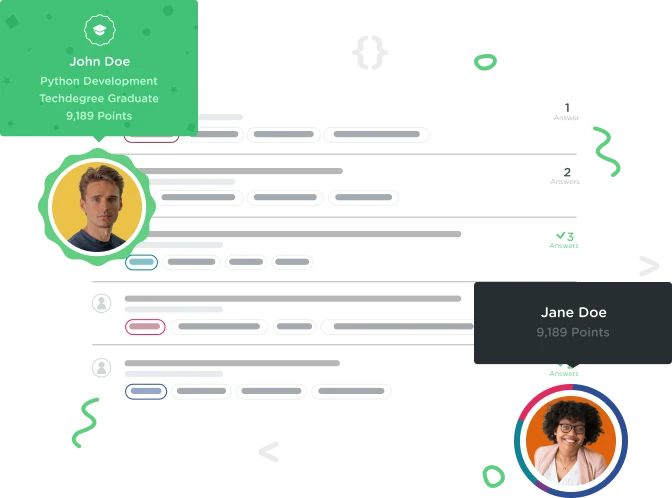
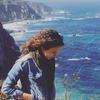
Deniz Kahriman
8,538 PointsPython Slices - Can you tell me what I'm doing wrong here? Thank you!
Last part of the challenge: Make a function and return items that have even indexes in a reverse order.
For instance, [1,2,3,4,5] should return [5,3,1]
def first_4(iterable): return iterable[0:4] def first_and_last_4(single): result = [ ] result = (single[0:4] + single[-4: ]) return result def odds(item): return item[1::2] def reverse_events(iterab): number = [] number = iterab[::] if len(number) %2 != 0: return number[:2:-1] else number.pop[] return number[:2:-1]
def first_4(iterable):
return iterable[0:4]
def first_and_last_4(single):
result = [ ]
result = (single[0:4] + single[-4: ])
return result
def odds(item):
return item[1::2]
def reverse_events(iterab):
number = []
number = iterab[::]
if len(number) %2 != 0:
return number[:2:-1]
else
number.pop[]
return number[:2:-1]
2 Answers
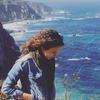
Deniz Kahriman
8,538 PointsThanks, Jay! Breaking the problem into steps was very helpful and way easier.
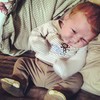
Jay Bennett
10,564 PointsMost welcome! It's so easy to get bogged down in the process of things. Knowing when to step back and reassess is definitely a skill I wish I'd picked up sooner. Feel free to message me any time about this stuff. I'm still learning, myself, but I love talking about it.
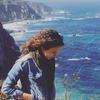
Deniz Kahriman
8,538 PointsThank you!! :)
Jay Bennett
10,564 PointsJay Bennett
10,564 PointsYou've got two syntax errors and the logic is wrong. Try to break the problem down. You'll want to, first, find the values at your even indexes and store them for reinsertion. Reverse their order. Then reiterate 'iterab' and reinsert them.
On line one, you're declaring an empty list. (Variable declaration in this way is unnecessary in Python. No harm in it, as far as I know though) On line two, you're copying the entire value of the parameter to that list. On line three, checking to see if the list contains an odd number of values. If so, you're returning everything in that list from it's finish to index 2 (non - inclusive) If not, you're attempting to remove and "return" the last item in the list. The second return will never fire because you'll either a) exit the function with a value from the pop() function or error on an empty list.
Also, don't forget the colon after your else branch and parenthesis after pop.