Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial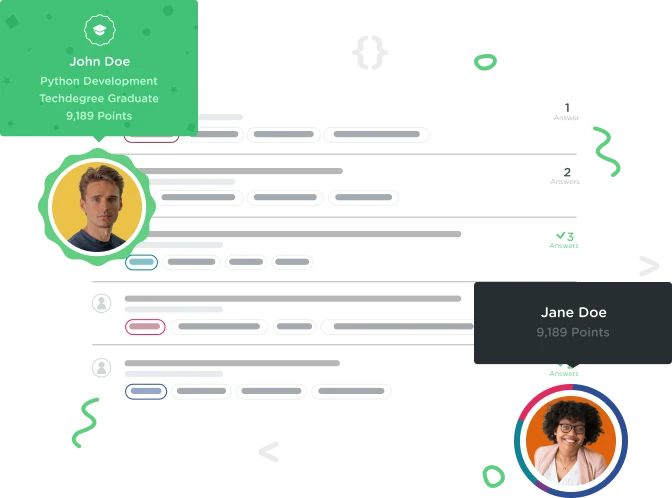
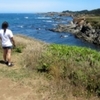
Nancy Melucci
Courses Plus Student 35,157 PointsPython string function "bound method" output instead of values.
I wrote a simple Circle class in Python with methods that work and produce correct values (I think). I need to write a str function to output the characteristics of the circle and when I call the attributes and method results I get the following output...
201.06192982974676 50.26548245743669 16 The circle has radius 8 with an area of <bound method Circle.area of <Circle.Circle object at 0x0000000002D1C518>>and a diameter of <bound method Circle.diameter of <Circle.Circle object at 0x0000000002D1C518>> and a circumference of <bound method Circle.circumference of <Circle.Circle object at 0x0000000002D1C518>>
How do I make that stop and replace it with the actual values? Code of class and instantiation follows...
Thank you.
import math
class Circle():
def __init__(self, r):
self.radius = r
def area(self):
return self.radius**2*math.pi
def circumference(self):
return 2*self.radius*math.pi
def diameter(self):
return 2*self.radius
def __str__(self):
return "The circle has radius " + str(self.radius) + " with an area of " + str(self.area) + "and a diameter of " + str(self.diameter) + " and a circumference of " + str(self.circumference)
from Circle import Circle
import math
c1 = Circle(8)
print(c1.area())
print(c1.circumference())
print(c1.diameter())
print(str(c1))
3 Answers
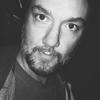
Chris Sederqvist
7,596 PointsAdd method calls:
def __str__(self):
return "The circle has radius " + str(self.radius) + " with an area of " + str(self.area()) + " and a diameter of " + str(self.diameter()) + " and a circumference of " + str(self.circumference())
This fixes the output.
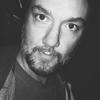
Chris Sederqvist
7,596 PointsThis formatting would appear with two decimal points:
def __str__(self):
return "The circle has radius {0:.2f} with an area of {1:.2f} and a diameter of {2:.2f} and a circumference of {3:.2f}".format(self.radius, self.area(), self.diameter(), self.circumference())
For the print statements:
print("%.2f" % c1.area())
print("%.2f" % c1.circumference())
print("%.2f" % c1.diameter())
Good luck!
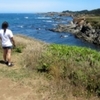
Nancy Melucci
Courses Plus Student 35,157 PointsFollow up question, if anyone is out there. Is there a way to format the diameter, area and circumference to two floating decimals?
Thanks.