Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial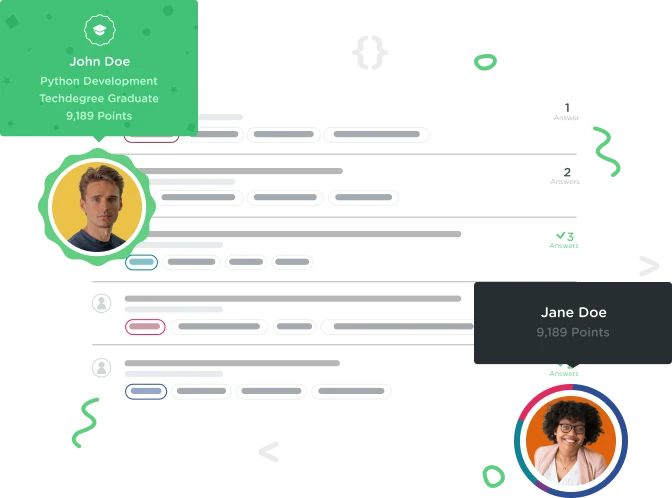
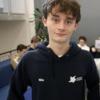
Mike Hansen
813 PointsPython won't spit out sys.exit message in try function
Hey! I'm currently playing around with Python trying to learn the language and I decided to take the end project, making a tickets app in cmd prompt in the course 'Python for beginners' into my own hands.
I am using the Try/except function at the beginning of the code as I want to prevent the user from actually buying more tickets than what's available. Everything is working excellent except for one of the sys.exit() functions.
Under the try: section I have an error message spitting out: "Woah! Slow down there, Cowboy! You're trying to buy too many tickets. We only have {} ticket(s) left.", however, when I exceed the amount available, which is 100 in this case, it just exits the cmd prompt, without actually spitting out the error message.
I also have a sys.exit function at the very end spitting out "okay, then." if you refuse to buy the tickets and that's working completely fine, so, why won't the first sys.exit function spit out the error as it's supposed to?
Here's the code:
#price in $
TICKET_PRICE = 10
tickets_remaining = 100
#Asking for username through input and assigning it to a new variable
name = input("Hello! What is your name? ")
#Saying hello to the user, including name, making it more personal
print("Welcome to the page, {}. There are currently {} tickets remaining.".format(name, tickets_remaining))
#Using the try/except function here as I want to prevent users from buying more than what's actually in stock. If the user exceeds the ticket_remaining they will be thrown out, else, continue
try:
quantity = int(input("How many tickets would you like to buy? "))
if quantity < tickets_remaining:
sys.exit("Woah! Slow down there, Cowboy! You're trying to buy too many tickets. We only have {} ticket(s) left.".format(tickets_remaining))
except:
subtotal = math.ceil(quantity * TICKET_PRICE)
answer = input("The subtotal is gonna be ${}, would you like to continue? (yes/no) ".format(subtotal))
if answer == 'yes':
sys.exit(("Sold to the buyer {} for ${}! Thanks for the purchase, {}, we hope that you enjoy the show.".format(name, subtotal, name)))
else:
sys.exit("Okay, then.")
2 Answers
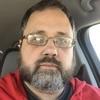
Mark Sebeck
Treehouse Moderator 37,776 PointsI wouldn't use a try block there. There is no error for it to fail on. You could use a a try block to stop them from entering letters or for a divide by 0. I would just use an else like this:
import sys
import math
#price in $
TICKET_PRICE = 10
tickets_remaining = 100
#Asking for username through input and assigning it to a new variable
name = input("Hello! What is your name? ")
#Saying hello to the user, including name, making it more personal
print("Welcome to the page, {}. There are currently {} tickets remaining.".format(name, tickets_remaining))
#Using the try/except function here as I want to prevent users from buying more than what's actually in stock. If the user exceeds the ticket_remaining they will be thrown out, else, continue
#try:
quantity = int(input("How many tickets would you like to buy? "))
if quantity > tickets_remaining:
sys.exit("Woah! Slow down there, Cowboy! You're trying to buy too many tickets. We only have {} ticket(s) left.".format(tickets_remaining))
else:
#except:
subtotal = math.ceil(quantity * TICKET_PRICE)
answer = input("The subtotal is gonna be ${}, would you like to continue? (yes/no) ".format(subtotal))
if answer == 'yes':
sys.exit(("Sold to the buyer {} for ${}! Thanks for the purchase, {}, we hope that you enjoy the show.".format(name, subtotal, name)))
else:
sys.exit("Okay, then.")
I think it also asks to make a loop to buy multiple tickets. Hopefully you will give that a try too. Good luck and keep at it!
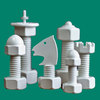
Steven Parker
230,274 PointsI'm not sure how you were seeing that final message. According to the documentation:
The sys.exit() function allows the developer to exit from Python. The exit function takes an optional argument, typically an integer, that gives an exit status. Zero is considered a “successful termination”.
Mike Hansen
813 PointsMike Hansen
813 PointsHey, of course. I changed up the entire code afterwards and found another way around it. But of course, I could've just removed the Try exception. Thanks!