Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial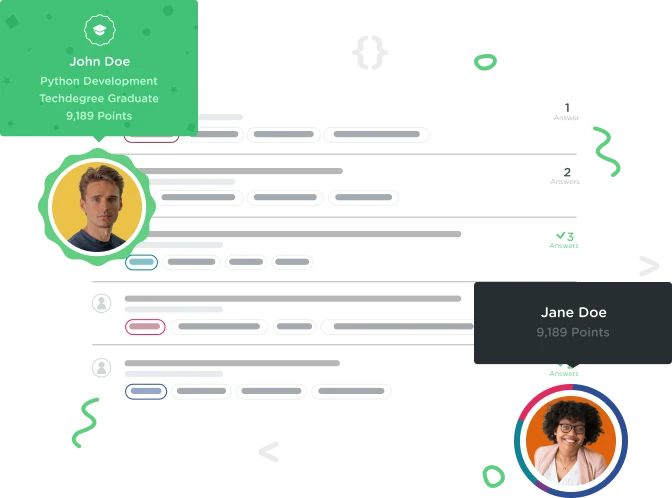

Robert Baucus
3,400 PointsPython: Word Count Challenge, using .count() and zip()
"""Alright, this one might be a bit challenging but you've been doing great so far, so I'm sure you can manage it.
I need you to make a function named word_count. It should accept a single argument which will be a string.
The function needs to return a dictionary.
The keys in the dictionary will be each of the words in the string, lowercased.
The values will be how many times that particular word appears in the string.
Check the comments below for an example.
E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
{'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
Lowercase the string to make it easier.
"""
#your previous attempt
def word_count(string):
dict = {}
split_string = ((string.lower()).split())
for word in split_string:
word_count = list(split_string.count(word))
dict = dict(zip(split_string, word_count))
print(dict)
word_count("The thing about counting things is the problem with keeping track of the things")
I am trying to create a dictionary from the output of using the split() method on the input argument zipped with the output of the count() method used on the same argument (string). Python tells me that the output of count() is not iterable, but I thought I had created a list of the counts for each world by the 'for' loop (iterating over each of the parts of split_sting)
I tried simply turning the output of the count() into a list, using list() but I fear that I simply have created a single number from the count(). I am totally turned around here.
Here is what Python3.6 has to say about it:
C:\Program Files\Python36\learningPython>python word_count.py Traceback (most recent call last): File "word_count.py", line 23, in <module> word_count("The thing about counting things is the problem with keeping track of the things") File "word_count.py", line 19, in word_count word_count = list(split_string.count(word)) TypeError: 'int' object is not iterable
1 Answer
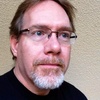
Chris Freeman
Treehouse Moderator 68,423 Pointsword_count
is being assigned in every iteration overwriting the previous value. Perhaps you mean for the dict
statement to be indented inside the for loop?
Post back if you need more help. Good luck!!!

Robert Baucus
3,400 PointsThank you. It works now. Just to make sure that I am getting this right: in the posted example (un-indented) the vale dict[word] = word_count is being reset after the 'for' loop closes , therefore the last time it overwrites the value for 'word_count' would be the last item iterated in the for loop, 'things' which gets assigned a count of 2.
The indented results in:
{'the': 3, 'thing': 1, 'about': 1, 'counting': 1, 'things': 2, 'is': 1, 'problem': 1, 'with': 1, 'keeping': 1, 'track': 1, 'of': 1}
because the 'for' loop is now iterating the creation of each key with dict[word] = word_count .
Funny how it seems so simple when you look at it a second time.
Robert Baucus
3,400 PointsRobert Baucus
3,400 PointsOK, I gave up on the zip() and I tried it using the dictionary properties, I got a somewhat better result:
Python does seem to count at least one of the words, but does not seem to iterate over all of them, Python's output:
C:\Program Files\Python36\learningPython>python word_count.py
{'things': 2}