Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial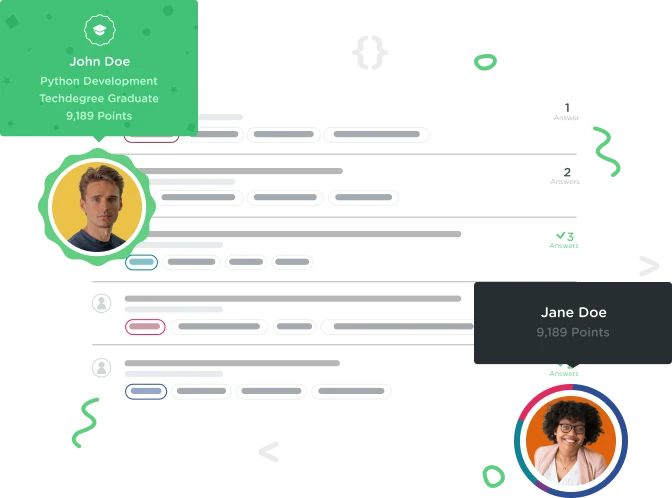
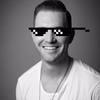
Seph Cordovano
17,400 PointsPython wordcount problem producing incorrect result?
I've gone over this quite a number of times, and pretty sure every angle is being covered as well as getting correct result in console. I know the return declaration isn't very pythonic but it's just for ensuring everything's correct for this specific problem.
The error is saying to make sure I'm lowercasing and splitting the words. Not only is that happening but I'm ensuring that it is a word and not a blank space with the if word:
conditional.
I've emailed support and they assure me this isn't a bug but I'm not seeing that so I'm turning to you guys to help me see if that's true.
Meme: 'this is a bug, prove me wrong' haha
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(words_string) -> dict:
words_dict = {}
words_list = words_string.lower().split(" ")
for word in words_list:
if word:
words_dict[word.strip()] = words_list.count(word)
return words_dict
1 Answer

andren
28,558 PointsThe error message you get asks you to make sure you are splitting on all whitespace. You are splitting on a space, which is a whitespace character, but not the only one. Tabs, line breaks, and various other characters also count as whitespace.
Conveniently enough the split
method will actually split on all whitespace by default if you do not pass it any arguments. So if you take your current code and simply remove the argument you pass to split
like this:
def word_count(words_string) -> dict:
words_dict = {}
words_list = words_string.lower().split()
for word in words_list:
if word:
words_dict[word.strip()] = words_list.count(word)
return words_dict
Then it will pass just fine.