Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial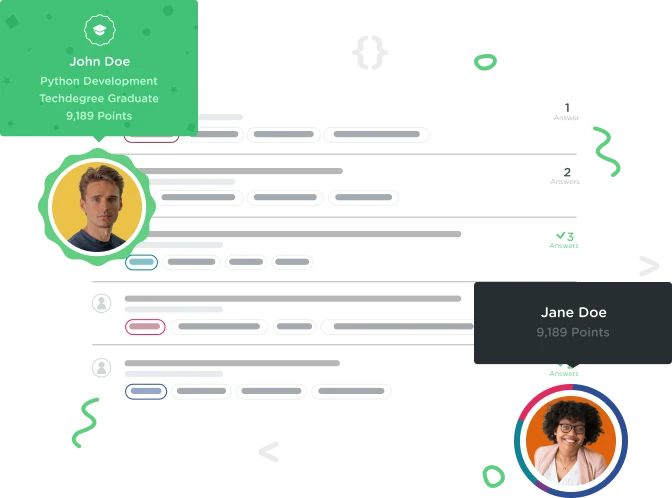

Joe Shaw
10,910 PointsQuerying with Linq - Partitioning Challenge 2/2
Hello , I have been beating my head against this for some time and getting very frustrated. I keep getting the error that my nextThreeBirds variable cannot contain birds from my firstThreeBirds variable but the code seems to be find. PLEASE HELP.
var birds = new List<Bird>
{
new Bird { Name = "Cardinal", Color = "Red", Sightings = 3 },
new Bird { Name = "Dove", Color = "White", Sightings = 2 },
new Bird { Name = "Robin", Color = "Red", Sightings = 5 },
new Bird { Name = "Canary", Color = "Yellow", Sightings = 0 },
new Bird { Name = "Blue Jay", Color = "Blue", Sightings = 1 },
new Bird { Name = "Crow", Color = "Black", Sightings = 11 },
new Bird { Name = "Pidgeon", Color = "White", Sightings = 10 }
};
var firstThreeBirds = birds.Take(3);
var nextThreeBirds = birds.OrderBy(b => b.Name.Length).Skip(3).Take(3);
4 Answers
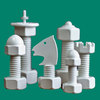
Steven Parker
229,785 Points
Don't re-order the list.
By changing the list order, your "next" three birds are not completely different from your "first" three.
Just get rid of the "OrderBy" — the challenge did not ask for that anyway.
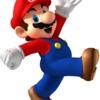
Terry McDuffie
12,786 Pointsvar birds = new List<Bird> { new Bird { Name = "Cardinal", Color = "Red", Sightings = 3 }, new Bird { Name = "Dove", Color = "White", Sightings = 2 }, new Bird { Name = "Robin", Color = "Red", Sightings = 5 }, new Bird { Name = "Canary", Color = "Yellow", Sightings = 0 }, new Bird { Name = "Blue Jay", Color = "Blue", Sightings = 1 }, new Bird { Name = "Crow", Color = "Black", Sightings = 11 }, new Bird { Name = "Pidgeon", Color = "White", Sightings = 10 } };
var firstThreeBirds = birds.Take(3);
var nextThreeBirds = birds.Skip(3).Take(3);

Sara Rena Anderson
15,045 Pointsvar birds = new List<Bird>
{
new Bird { Name = "Cardinal", Color = "Red", Sightings = 3 },
new Bird { Name = "Dove", Color = "White", Sightings = 2 },
new Bird { Name = "Robin", Color = "Red", Sightings = 5 },
new Bird { Name = "Canary", Color = "Yellow", Sightings = 0 },
new Bird { Name = "Blue Jay", Color = "Blue", Sightings = 1 },
new Bird { Name = "Crow", Color = "Black", Sightings = 11 },
new Bird { Name = "Pidgeon", Color = "White", Sightings = 10 }
};
var firstThreeBirds = birds.Take(3);
var nextThreeBirds = birds.Skip(3).Take(3);

Kevin Gates
15,052 PointsYour solution provides an "OrderBy" but the question didn't ask for that. It is only needing to skip the first 3 before you take the next 3.
var birds = new List<Bird>
{
new Bird { Name = "Cardinal", Color = "Red", Sightings = 3 },
new Bird { Name = "Dove", Color = "White", Sightings = 2 },
new Bird { Name = "Robin", Color = "Red", Sightings = 5 },
new Bird { Name = "Canary", Color = "Yellow", Sightings = 0 },
new Bird { Name = "Blue Jay", Color = "Blue", Sightings = 1 },
new Bird { Name = "Crow", Color = "Black", Sightings = 11 },
new Bird { Name = "Pidgeon", Color = "White", Sightings = 10 }
};
var firstThreeBirds = birds.Take(3);
var nextThreeBirds = birds.Skip(3).Take(3);