Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial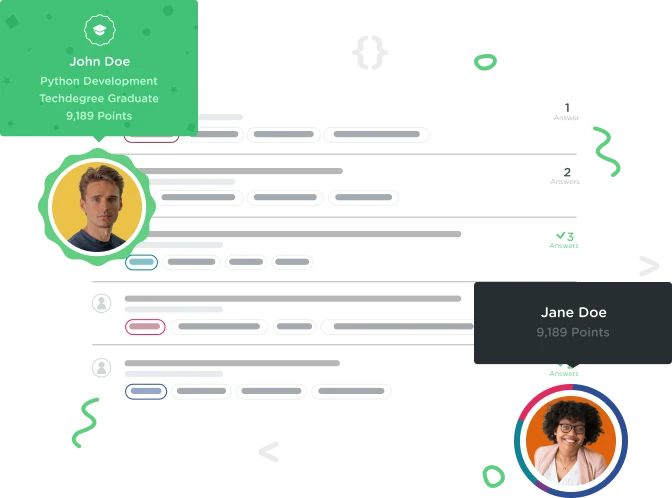

Julio Mayorga
648 Points.querySelector ("") not working
I am stuck in the following question of Interactive web pages using javascript. It is asking that listItems should be requesting the children of navigation. So, I used .querySelector("");
My code looks like this:
var listItems = navigation.querySelector("li");
Because the li tags are children of the ul element who's ID is navigation.
//Select the naviagation
var navigation = document.getElementById("navigation");
//Select all listItems from the navigation
var listItems = navigation.querySelector("li");
//When a navigation link is pressed
var linkListener = function() {
console.log("Listener is clicked!");
}
var bindEventsToLinks = function(listItem) {
//Select the anchor
var anchor = listItem;
//Bind the linkListener to the anchor element (a)
anchor.onclick = linkListener;
}
for(var i = 0; i < listItems.length ; i++) {
bindEventsToLinks(listItems[i]);
}
<!DOCTYPE html>
<html>
<head></head>
<body>
<ul id="navigation">
<li>
<a href="#home">Home</a>
</li>
<li>
<a href="#about">About</a>
</li>
<li>
<a href="#contact">Contact</a>
</li>
</ul>
<p>A few of my favourite things:</p>
<ul>
<li>
Rain drops on roses
</li>
<li>
Whiskers on kittens
</li>
<li>
Brown paper packages wrapped up with string
</li>
</ul>
<script src="app.js"></script>
</body>
</html>
2 Answers
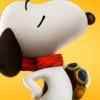
Salman Akram
Courses Plus Student 40,065 PointsHi Julio,
You can use .children
instead .querySelector("li")
var listItems = navigation.children;
-Salman
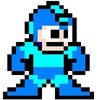
Robert Richey
Courses Plus Student 16,352 PointsHi Julio,
// use either this
var listItems = navigation.children;
// or this
var listItems = navigation.querySelectorAll("li");
From W3Schools
The querySelector() method returns the first element that matches a specified CSS selector(s) in the document.

Julio Mayorga
648 PointsOh ok. So, to understand: I can use the .querySelectorAll if I wish to select all of the li tags? The .querySelector only selects the first li tag? And the .children selects all of the parent's children?