Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial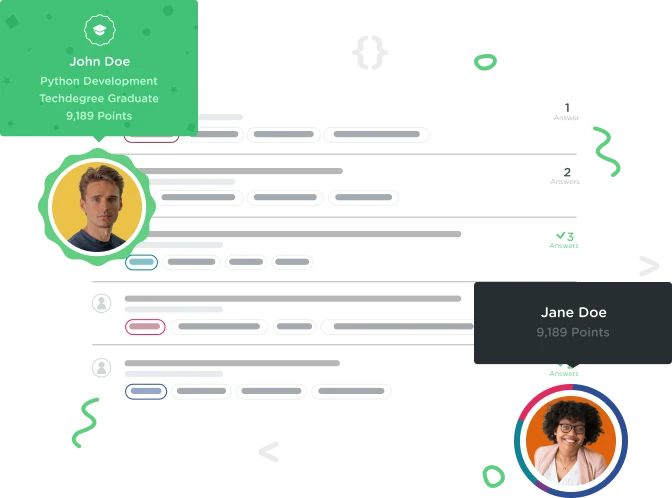

Don Noray
19,238 Pointsquestion
Javascript Functions
Challenge task 1 of 1
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in. Error: Bummer! If I pass in an array in to 'arrayCounter' of '1,2,3' I get undefined and not the number 3.
I have been trying to solve this problem for over a week to no avail. Can I please get some help as to what I'm doing wrong?
function arrayCounter(arr) {
if (typeof arr === Array) {
return arr.length;
}
else if (typeof arr === 'string' || arr === undefined || !isNaN(arr) ) {
return 0;
}
}
3 Answers
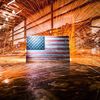
Andrew Shook
31,709 PointsDon, the problem here is that you have run into a JavaScript idiosyncrasy. In JavaScript, typeof array() will return "object" not array, see explanation of typeof. Near the bottom is a code block, look at line 34. In reality the question should be asking you to using Array.isarray();
If you replace the Array in your conditional with 'object' you should be good to go. Also, on a side note, you don't need the else if statement at the bottom of your code. Since the question asks you to only return the length if it is an array, you could just use:
if(something is true){
return some value;
}else{
return some other value;
}
or you could do:
if(something){
return some value;
}
return some other values;

James Barnett
39,199 PointsThe easiest way to check if a variable is an array
is to see if it's an instance of the Array constructor
.
Use:
if (arr instanceof Array) { }
Instead of:
if (typeof arr === Array) { }

Don Noray
19,238 PointsThanks James!

Don Noray
19,238 PointsThanks a lot! It worked. Thanks for the info on the else if.