Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial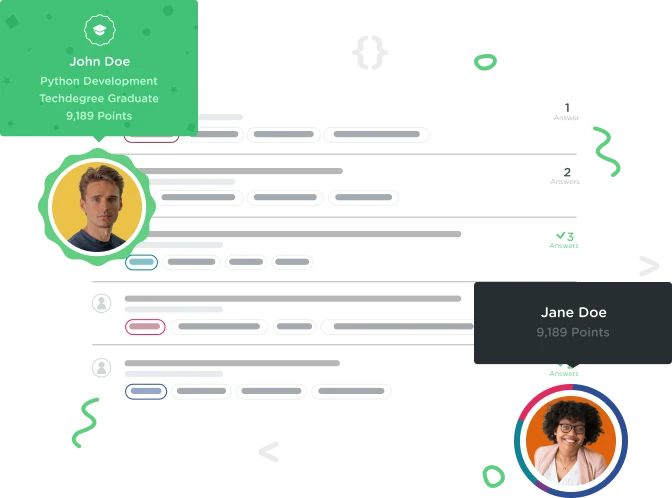

Kyle Baker
1,884 PointsQuestion about adding style via getStyleClass()
So I'm confused about this technique of assigning a style to the VBox. I don't see the logic in it. Using the Pomodoro app as an example, if we insert 'kind' (which would be FOCUS or BREAK) into the style sheet using:
container.getStyleClass().add(kind.toString().toLowerCase());
wouldn't that just be inserting 'focus' into the style sheet? How is that a readable form of a class? How does it know what to do with 'focus' if it's not in the .focus {} format? And regardless, if we're removing all 'kinds' from the style sheet before we do this with:
container.getStyleClass().remove(kind.toString().toLowerCase());
how does it know any of the properties of the .focus class if they've all been removed? If my question is difficult to understand it's because I'm having difficulty understanding...thanks for understanding!
package com.example;
import com.example.model.Brand;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
public class Controller {
@FXML
private VBox container;
public void handlePlay(ActionEvent actionEvent) {
System.out.println("[MUSIC PLAYING] - I shot the sheriff, but I didn't shoot the deputy");
}
public void switchBrand(Brand brand) {
clearBrandStyles();
addBrandStyles();
}
public void addBrandStyles(Brand brand) {
container.getStyleClass().add(brand.toString().toLowerCase());
}
public void clearBrandStyles() {
for (Brand brand : Brand.values()) {
container.getStyleClass().remove(brand.toString().toLowerCase());
}
}
public void handleBrandSwitch(ActionEvent actionEvent) {
// Get a handle on the calling button
Button btn = (Button) actionEvent.getSource();
// Get access to the brand like Brand.APPLE by name
Brand brand = Brand.valueOf(btn.getText().toUpperCase());
switchBrand(brand);
}
}
/* Brand settings */
.sony {
-fx-background-color: black;
}
.jvc {
-fx-background-color: red;
}
.apple {
-fx-background-color: silver;
}
.coby {
-fx-background-color: gray;
}
4 Answers

Seth Kroger
56,413 PointsThe stylesheet (the CSS) doesn't get altered at all. The "style class" is a list of classes that you should apply the styles in the stylesheet for. By adding the focus class to the container, you are saying that any CSS styles with the selector ".focus" (such as ".focus { ... }" and others) should be applied to those elements.
This works almost identical to HTML/CSS for web pages. If you had a div with the class of "container" and wanted to add "focus" it be like changing this:
<div class="container">
...
</div>
<div class="container focus">
...
</div>
If you are unfamiliar with HTML and CSS I suggest looking at the How to Make a Website course for a basic overview: https://teamtreehouse.com/library/how-to-make-a-website It will help tremendously in understanding how JavaFX uses CSS.
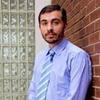
Zach Freitag
20,341 PointsSpoiler Alert!
package com.example;
import com.example.model.Brand;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
public class Controller {
@FXML
private VBox container;
public void handlePlay(ActionEvent actionEvent) {
System.out.println("[MUSIC PLAYING] - I shot the sheriff, but I didn't shoot the deputy");
}
public void switchBrand(Brand brand) {
clearBrandStyles();
container.getStyleClass().add(brand.getDisplayName().toLowerCase());
}
public void clearBrandStyles() {
for (Brand brand : Brand.values()) {
container.getStyleClass().remove(brand.toString().toLowerCase());
}
}
public void handleBrandSwitch(ActionEvent actionEvent) {
// Get a handle on the calling button
Button btn = (Button) actionEvent.getSource();
// Get access to the brand like Brand.APPLE by name
Brand brand = Brand.valueOf(btn.getText().toUpperCase());
switchBrand(brand);
}
}

Juan Chavez
2,995 PointsAdd brand to addBrandStyles method
public void switchBrand(Brand brand) {
clearBrandStyles();
addBrandStyles(brand);
}
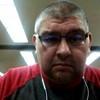
micram1001
33,833 PointsThanks, the above info is helpful. Michael