Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial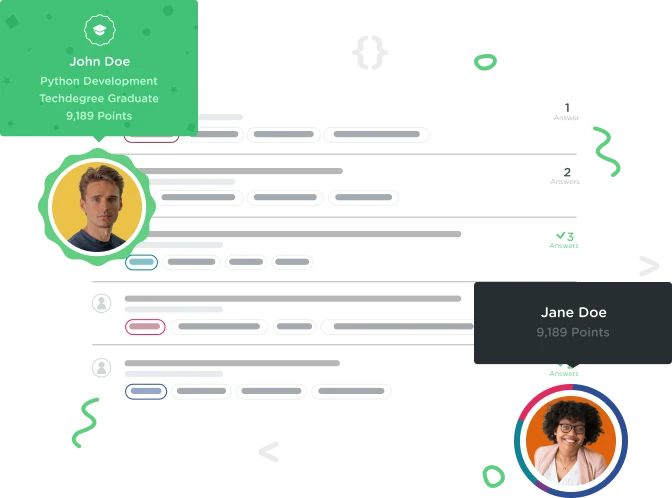

Ibrahim Adeosun
2,973 PointsQuestion about default values in java
I have a problem that I believe is being caused by default values for variables in java.
public class Test {
private static int I;
public static void main(String args[]) {
System.out.println("Value of integer i is : " + obj);
}
}
When I do that it prints "Value of integer i is : 0"
public class Test {
public static void main(String args[]) {
int i;
System.out.println("Value of object i is : " + i);
}
}
When I do this it doesn't run it gives an error. I don't know why but I understand that when a variable is declared whether a reference variable or a primitive variable java sets a default value if they are not initialised and in the case of integers it is set to 0 which is what is happening in the first example but not the second. Why?
2 Answers

Ibrahim Adeosun
2,973 PointsOk, so I finally found an answer to this. So in java, there are three types of variables. namely: local, static/class and instance. Both class and instance variables get default values but local variables do not. Local variables are variables declared in methods or constructors and in the example I gave the variable that had no default value was the one declared within the main method.
For more - https://www.tutorialspoint.com/java/java_variable_types.htm

saykin
9,835 PointsThis is just a behavior of Java, when a variable is declared outside a method, it's a member of that class and get's a default value. This is done (I assume) because a variable field in the class can be used by all methods within that class and potentially by other classes too. So to avoid issues, Java gives them a default value. In Integer's case, 0.
When it's inside a method, that variable is usually used for local use within that method and needs to be initialized. This variable (note: not the value it's pointing to) cannot be used outside of this method.
Generally speaking though, you should always give them a value, even if that value is 0. It makes the code easier to understand and you avoid potentially weird behavior in your code.