Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial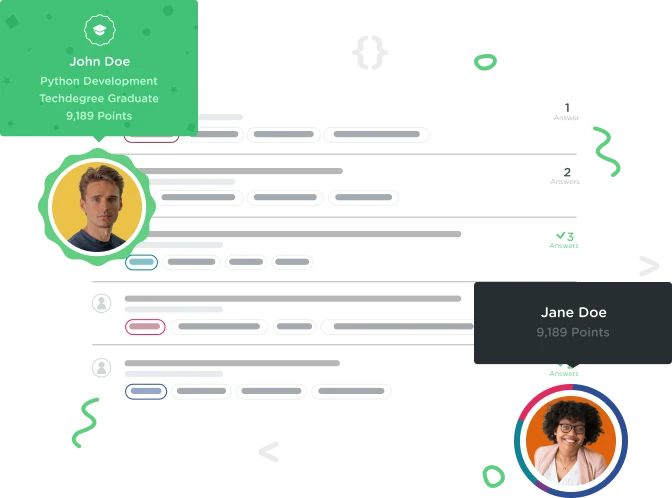

Greg Schudel
4,090 PointsQuestion about DOM minipulation
I have some basic questions about some specific code.
The JS IS this, please see specific questions labeled with 'Q: '
const descriptionButton = document.querySelector('button.description');
const descriptionP = document.querySelector('p.description');
const descriptionInput = document.querySelector('input.description');
/* this is ES6 version of a function (now an arrow function) we are grabbing the varaible we just defined above and throwing
a EventListener method on it
*/
descriptionButton.addEventListener("click", () => {
descriptionP.innerHTML= descriptionInput.value + ':';
descriptionInput.value = ''; //************************* Q: why the heck is this here?
});
// the above looks innaccurate from the arrow functions I have seen
/******************************** Q:Shouldn't it be like this? Right?
//ES6 ARROW FUNCTION VERSION
var description = ('click') => {
descriptionP.innerHTML= descriptionInput.value + ':';
descriptionInput.value = '';
});
*/
The full code is here in CodePen: https://codepen.io/webwatchman/pen/oeOEEG
3 Answers
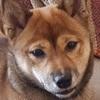
Katie Wood
19,141 PointsI just saw two Q's in there - let me know if I missed one!
First Q: I believe that line is there so that when it saves the input, it will clear the field. Think of typing a text message - you're typing an input, and once you've entered it, it automatically clears it so you can enter something else if you want.
Second Q: The arrow function is used correctly in the example above - I think what might have caused the confusion is that the arrow function is being created inside a function call - namely the addEventListener() function. The arrow function is the second parameter, and does not need to take the click as a parameter because the addEventListener() function is already told to listen for a click. That seems like a weird sentence, so I'm going to break that down a little:
//replaced the code with what the actual parameters are
descriptionButton.addEventListener(listenerType, whatToDo);
So, "click" is passed into addEventListener already, as the type. The arrow function is only there to tell it what to do when it detects the click, since addEventListener is taking care of the rest. So, we get:
descriptionButton.addEventListener("click", () => { //create a listener on the descriptionButton, to execute the arrow function when it detects a click on the button
descriptionP.innerHTML= descriptionInput.value + ':'; //stick a colon on the end
descriptionInput.value = ''; //clear the input field so it's easy to enter more values
});
In the example you gave below the code, you're actually setting the function to a variable, which may not be what you want. What you're doing there is creating your own function, stored to a variable called 'description'. That wouldn't execute unless you called it, which you could do, but you would still need an event handler to use it on a button click. Passing 'click' when you're creating a function also won't work the way it does in addEventListener. The addEventListener function is already defined in the JavaScript library, which means it's already expecting an event type as a parameter, which is why passing "click" works. Arrow functions are for creating your own functions, so you would have to define the type of input required, not a specific value.
Does that make sense?

Greg Schudel
4,090 PointsAnother question,
What is the value method?
descriptionButton.addEventListener("click", () => { // I get this now!
descriptionP.innerHTML= descriptionInput.value + ':';
descriptionInput.value = ''; // what is the value method? Don't see anything online for that?
});
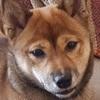
Katie Wood
19,141 PointsHey again,
In this example, descriptionInput represents a text input field. 'Value' is an attribute of that field representing the text in the box. Here, we're setting the box's value to nothing, making the field blank. For more info on the attribute itself and other input field attributes, try here and here!

Greg Schudel
4,090 PointsGreat! Makes so much sense!
In the example you gave below the code, you're actually setting the function to a variable, which may not be what you want.
Actually. that technique works perfectly well for what I want. I need that addEventListener tacked onto that variable as a method so whenever you click the description in this HTML, the title won't go blank before you type in anything.
This is my HTML doc
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<div class="list">
<p class="description">Things that are purple:</p>
<input type="text" class="description" value="Insert Your answer here">
<button class="description"> Change List description </button>
<ul>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
</div>
<script src="app_v3.js"></script>
</body>
</html>
Run this code without that variable and you'll have the title erase immediately. That's not what I want. Experiment with my CodePen (URL IS ABOVE) if you want to see what I mean. Run this JS in the editor
const descriptionButton = document.querySelector('button.description');
const descriptionInput = document.querySelector('input.description');
const paragraph = document.querySelector('p.description');
addEventListener ('click', () => { //no variable here
paragraph.innerHTML = descriptionInput.value + ':';
descriptionInput.value = '';
});
and compare that with this:
const descriptionButton = document.querySelector('button.description');
const descriptionInput = document.querySelector('input.description');
const paragraph = document.querySelector('p.description');
descriptionButton.addEventListener ('click', () => {// variable added from above 'descriptionButton' declaration
paragraph.innerHTML = descriptionInput.value + ':';
descriptionInput.value = ''; // this uses the value attribute, this defines.
// The value attribute is not a method per say, its an attribute of the HTML that you have control
// without having to declare it in a variable. ITS PREDEFINED!
});
See what I mean?
Greg Schudel
4,090 PointsGreg Schudel
4,090 PointsAhhh thank you! Off I go to play with javascript!
Katie Wood
19,141 PointsKatie Wood
19,141 PointsAwesome - have fun!