Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial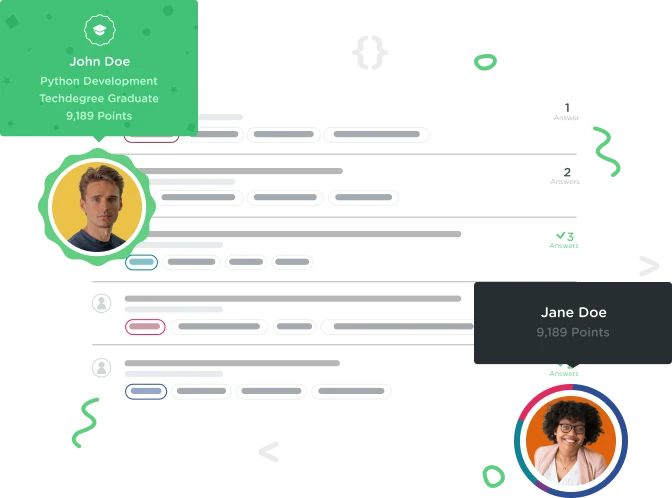

dlpuxdzztg
8,243 PointsQuestion about exercise 2...
Hello, I completed the challenge for this video, but i'm still having doubts about it...
I'm not sure what the code I wrote for the challenge actually does, mainly because i'm still a little confused on type casting and superclasses and how it all works...
Help is appreciated!

Simon Coates
28,694 PointsI tried code similar to Jeremy Hill's sample code, and demonstrated that java wouldn't let me compile with a conversion of String to int. The conversion is possible in other languages, but java is pretty strict.
2 Answers

Simon Coates
28,694 PointsA lot of the time Java knows what the class is. It can accept a subclass where a superclass is expected, and in that circumstance is safe to call any superclass methods on the subclass (this methods will have been defined in the parent, if not overridden in the child). You cast when java need to be told that an object is a specific type (it's not already implicit). An example might be if an object is dynamically a string, but received as an Object type. Java can't know that the string methods are safe to be called, so you might need to cast it into a string to tell java, it can be used as one. (most of the time you shouldn't need to tell java about the object if you design well). I think the casting terminology is also used when converting primitives, but in that instance, it's a real conversion. Casting on an object doesn't change the object.

Simon Coates
28,694 PointsIn the following, java only knows that the objects in some are of person type (fyi student is a subtype). before it lets you compile with a call to addClass( a method on the student subtype), it has to be told that the Person is actually a Student to compile. If you tell it the object is a student, but it isn't, you will get a runtime error (hence the need for instanceof).
public static void main(String[] args)
{
List<Person> some = new ArrayList<Person>();
some.add(new Student());
some.add(new Person());
for(Person p: some) {
if(p instanceof Student){
//p.addClass();//will fail compile
((Student) p).addClass();
}
//((Student) p).addClass(); // compiles but crashes for the student.
}
}
The object doesn't actually change types with objects.

Simon Coates
28,694 PointsRegarding primitive conversion, you can see the received value is different to the original
public static void main(String[] args)
{
double x = 0.5;
//int y = x;//won't compile
int y =(int) x;
System.out.println("x: "+x+", y:"+y);
String z = "6";
//int zz = (int) z; //cannot cast from string to int
int zz = Integer.valueOf(z);
System.out.println("z: "+z+", zz:"+zz);
}
produces: x: 0.5, y:0 z: 6, zz:6

dlpuxdzztg
8,243 PointsSo casting is basically converting?

Simon Coates
28,694 PointsNo, casting is only converting with primitives (int, double etc). With objects, it just tells the code that you know more information about an object. For example, a method has an Object o, which you happen to know is a String. If you String aString = (String) o; then following that, you can write code that uses o's nature as a string (eg string methods). Otherwise, Java only accepts code that thinks o is an object. aString and o are both the exact same object, referring to the exact same space in memory. (does that help, or have i made things worse?)

dlpuxdzztg
8,243 PointsOkay, Thanks!
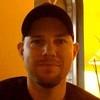
Jeremy Hill
29,567 PointsYea my bad, I always use the parseInt() method, but I always thought it would let you do it that way. I know that you can do it with objects any how. Java does seem to be more restrictive when it comes to type casting it seems.

Simon Coates
28,694 Pointscasting behaviour is a nightmare. I just remember not to trust it. My motto is "Live in fear".
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsHere is a small example of type casting:
When the compiler expects Strings from the user and if the user inputs the number 7 it will automatically be of type String; now one way to convert it would be to use a type cast. Like this:
String inputAsString = scanner.next();
int userInput = (int)inputAsString;
The int in parenthesis is where the type casting takes place.