Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial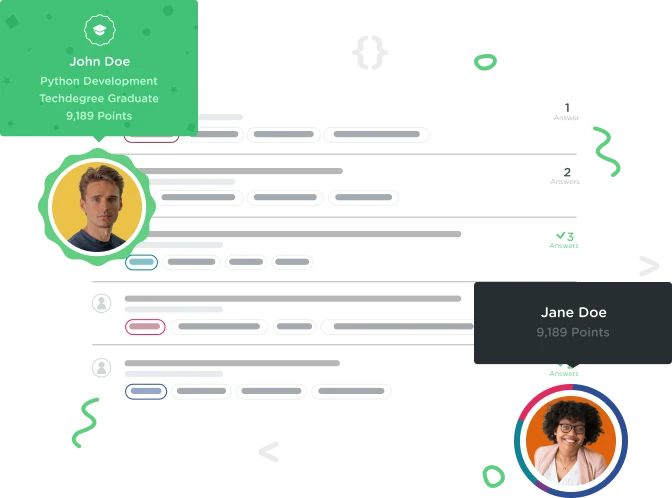

Luke Maschoff
820 PointsQuestion about functions using lists
OK, so when Craig first set the display_wishlist function, with the perimeters of (display_name, wishes), i'm confused on how he got the values of each.
def display_wishlist(display_name, wishes):
print(display_name + ":")
suggested_gift = wishes.pop(0)
print("=====>", suggested_gift, "<=====")
for wish in wishes:
print("* " + wish)
print()
On the second line, where is he getting the display_name from? Also, where is he getting the value of wishes? Also, what is with the print() function, with nothing inside it?
Here is the rest of the code if that helps:
books = [
"Learning Python: Powerful Object-Oriented Programming - Mark Lutz",
"Automate the Boring Stuff with Python: Practical Programming for Total Beginners - Al Sweigart",
"Python for Data Analysis - Wes McKinney",
"Fluent Python: Clear, Concise, and Effective Programming - Luciano Ramalho",
"Python for Kids: A Playful Introduction To Programming - Jason R. Briggs",
"Hello Web App: Learn How to Build a Web App - Tracy Osborn",
]
video_games = [
"The Legend of Zelda: Breath of the Wild",
"Splatoon 2",
"Super Mario Odyssey",
]
def display_wishlist(display_name, wishes):
print(display_name + ":")
suggested_gift = wishes.pop(0)
print("=====>", suggested_gift, "<=====")
for wish in wishes:
print("* " + wish)
print()
display_wishlist("Books", books)
display_wishlist("Video Games", video_games)
3 Answers

Luke Maschoff
820 PointsOk, I think i get it, so the list called books, flows into the display_name, and the type of book goes into wishes?
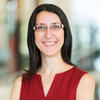
Louise St. Germain
19,424 PointsThat's almost right! You're right in that values from elsewhere flow into display_name and wishes. However, display_name gets the first thing in the function call, which is literally just the string "Books" (you can tell that it is a string and not the name of a variable because of the quotation marks ""). So inside the function, display_name = "Books".
wishes gets the second thing in the function call, which is the list books (this is a variable name) - and you can see the books list get defined at the very beginning of the program:
books = [
"Learning Python: Powerful Object-Oriented Programming - Mark Lutz",
"Automate the Boring Stuff with Python: Practical Programming for Total Beginners - Al Sweigart",
"Python for Data Analysis - Wes McKinney",
"Fluent Python: Clear, Concise, and Effective Programming - Luciano Ramalho",
"Python for Kids: A Playful Introduction To Programming - Jason R. Briggs",
"Hello Web App: Learn How to Build a Web App - Tracy Osborn",
]
So inside the function, once books has been passed to wishes, wishes contains the same list as books, and would look like this:
wishes = [
"Learning Python: Powerful Object-Oriented Programming - Mark Lutz",
"Automate the Boring Stuff with Python: Practical Programming for Total Beginners - Al Sweigart",
"Python for Data Analysis - Wes McKinney",
"Fluent Python: Clear, Concise, and Effective Programming - Luciano Ramalho",
"Python for Kids: A Playful Introduction To Programming - Jason R. Briggs",
"Hello Web App: Learn How to Build a Web App - Tracy Osborn",
]
The second time you call function, (last line of the program) different values are passed to it:
display_wishlist("Video Games", video_games)
So the second time around, display_name gets the string "Video Games" (again, literally the hard-coded string "Video Games" and not a variable name), and wishes gets the list that's in video_games, which was also defined near the beginning of the program.

Luke Maschoff
820 PointsOK, one more question though, it says print(display_name + ":") on one section, where does the display_name come from? Its no where else in the code?
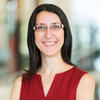
Louise St. Germain
19,424 Pointsdisplay_name also appears in the line directly above that, and that's where it's defined. It's one of the parameters defined for that function, which gets the string (like "Books") that we talked about earlier - the value for that comes from elsewhere and is passed to the function.

Luke Maschoff
820 PointsOk I get it, thanks!
Louise St. Germain
19,424 PointsLouise St. Germain
19,424 PointsHi Luke,
Simple things first: print() just prints a blank line, so he's using it to create some white space in the output.
For the parameters of display_wishlist, it sounds like there's a bit of confusion about how the function parameters work. If I'm wrong, you can skip the rest of this! :-)
Let's look at your second block of code, since we can see the whole flow of the program more easily.
At the top, you see the lists of values get assigned to the variables books and video_games. Next, you see the function definition for display_wishlist, which includes everything you put in your first block of code.
After that, at the end of the second block of code, you see two calls to this display_wishlist function, with two different sets of values.
The important thing to note in this overall flow is that the code for display_wishlist does not run until it called (like you see in those last two lines of code). If your whole program looked like your first block of code and had nothing else, the program would do nothing when you ran it because there is a function but no call that tells it to actually run.
When you call the display_wishlist function (toward the bottom of your second block of code), you include values which correspond to the defined parameters.
Let's look at the format of the parameters for the function definition:
Compare this to the first call:
When you do a function call, the arguments ("Books", books) provide the values for the parameters (display_name, wishes) So you can imagine it's automatically doing the following internally:
The next time you call the function, with different values, those different values will be passed into the function. That way you can kind of customize the info every time the function runs.
I hope this helps a bit.