Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial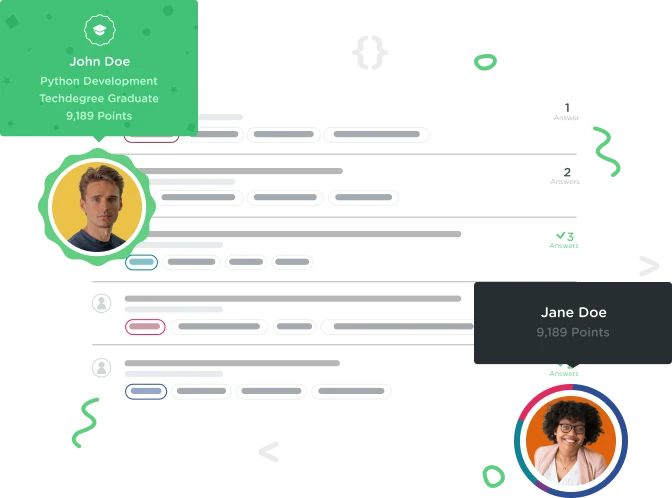

Shelby Kessel
Courses Plus Student 1,482 PointsQuestion About Getting Json Data Code Challenge
Hello I was wondering if anyone could help me with the last part of the "Getting JSON Data from an HTTP Request" it's under "Build a Blog Reader Android App". The task is "Finally, set the 'responseData' String variable with the data in 'charArray'. Convert it using the String constructor that takes a char array as its parameter." String responseData ="";
try { URL treehouseUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=20"); HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection(); connection.connect(); int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
InputStream inputStream = connection.getInputStream();
Reader reader = new InputStreamReader(inputStream);
char[] charArray = new char[connection.getContentLength()];
reader.read(charArray);
String responseData = new String(charArray);
}
I have tried multiple things on "Line 1" for the "declaration" but I keep getting it wrong, I have tried String responseData = "charArray"; I tried String responseData = new String(charArray); just to see if that might work, and some others but they have all been wrong, the error message I keep getting is "Compilation Error! Make sure you are setting 'responseData' correctly inside the 'if' block using 'new String()' with 'charArray' as the parameter. Note: 'responseData' is declared on line 1.", I understand having the String constructor part in the "if block" but I'm not sure what to declare, so If someone could help me I would greatly appreciate it :).
5 Answers

Ernest Grzybowski
Treehouse Project ReviewerYou entered:
String responseData = new String(charArray);
The error message treehouse gives you is:
Bummer! Compilation Error! Make sure you are setting 'responseData' correctly inside the 'if' block using 'new String()' with 'charArray' as the parameter. Note: 'responseData' is declared on line 1.
Pay close attention to the end of the error message from treehouse. They give you a note and the "note" pretty much sums up your problem.
You were trying to declare the string again even though it was declared in line 1. This is the complete code:
String responseData = "";
try {
URL treehouseUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=20");
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// Code to read InputStream goes here!
InputStream input = connection.getInputStream();
Reader reader = new InputStreamReader(input);
char[] charArray = new char[connection.getContentLength()];
reader.read(charArray);
responseData = new String(charArray);
}
}
catch (MalformedURLException e) {
Log.e(TAG, "MalformedURLException caught!", e);
}
catch (IOException e) {
Log.e(TAG, "IOException caught!", e);
}

Ernest Grzybowski
Treehouse Project ReviewerNo need to feel dumb. Now you'll never make the same mistake ever again. The trick was really understanding the question:
Finally, set the 'responseData' String variable with the data in 'charArray'. Convert it using the String constructor that takes a char array as its parameter." String responseData ="";
Set the responseData string variable? Not create it? Your eyes should look up and see if there has already been one created. Which there was.
Keep coding!

Shelby Kessel
Courses Plus Student 1,482 PointsWow lol, I feel kinda dumb now. Thanks for the help Ernest!

Riley MacDonald
2,542 PointsI am having trouble with this task as well. Here is my code:
String responseData = new String(charArray);
try {
URL treehouseUrl = new URL("http://blog.teamtreehouse.com/api/get_recent_summary/?count=20");
HttpURLConnection connection = (HttpURLConnection) treehouseUrl.openConnection();
connection.connect();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
InputStream inputStream = connection.getInputStream();
Reader reader = new InputStreamReader(inputStream);
char[] charArray = new char[connection.getContentLength()];
reader.read(charArray);
}
}
catch (MalformedURLException e) {
Log.e(TAG, "MalformedURLException caught!", e);
}
catch (IOException e) {
Log.e(TAG, "IOException caught!", e);
}

Riley MacDonald
2,542 PointsCan anyone help?

Ray Hayes
3,821 PointsYou are missing the last step which is to convert your array of characters from charArray into a string array using the String(); method. Ernest pretty much summed it up.