Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial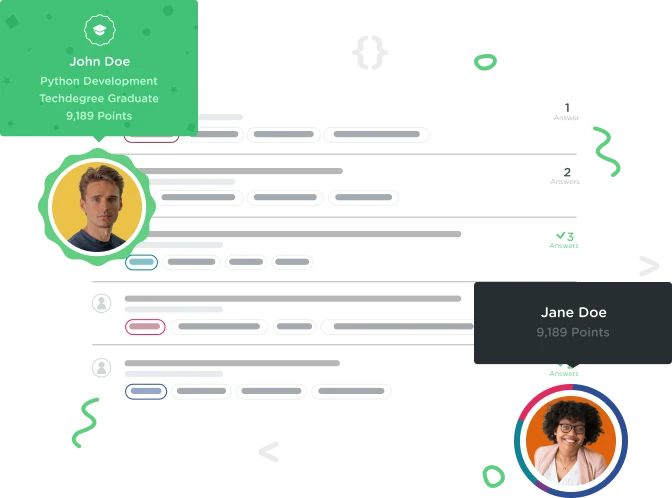

Mark Roudebush
901 PointsQuestion about my alternative solution
I see the comments are asking for the solution to work in a specific order, but I got the same result with a different approach. My question is outside of this challenge, would this alternative approach be wrong in any way or not sophisticated etc.? Thanks for any feedback.
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
if number % 3 == 0 and number % 5 == 0:
print ("Hey, {}! \nThe number {}... \nis a FizzBuzz number.".format(name, number))
elif number % 3 == 0:
print ("Hey {}! \nThe number {}... \nis a Fizz number. ".format(name, number))
elif number % 5 == 0:
print ("Hey {}! \nThe number {}... \nis a Buzz number. ".format(name, number))
if number % 3 != 0 and number % 5 != 0:
print ("Hey {}! \nThe number {}... \nis neither a fizzy or a buzz number.".format(name, number))

Abhinandan vig
445 PointsHey i went through the same approach but instead of quoting the format line again and again you should have written it before the if statement, that might make the program look neat otherwise it is fine. NO offence ! Here check mine : name = input("Please enter your name: ") number = int(input("Please enter a number: "))
print("hey", name) print("Your no", number)
if number%3 == 0 and number%5 == 0: print("is a fizzBuzz") elif number%3 == 0: print("is a fizzz") elif number%5 == 0: print("buzz") else: print(" is neither")
3 Answers

Mark Roudebush
901 PointsThanks Alex Koumparos , I fixed it

Mark Roudebush
901 PointsThanks, Abhinandan vig !!

Rana Olk
235 PointsIm not sure I understand the markdown cheatsheet or what its for, but here goes.. .This was my alternative, and I just want to ask, how do we know which code is better when there are different ways to code? Or is it whichever way is shortest, that's the best?
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
print(name)
print(number)
if number%3==0 and number%5==0:
print("is a fizzbuzz number")
elif number%3==0:
print("is a fizz number")
elif number%5==0:
print("is a buzz number")
else:
print("is neither a fizz nor a buzz number")

mariem
5,586 PointsI think both of your alternatives are fine. The thing is that we were asked to create a variable for is_fizz and is_buzz. This way, we could use those variables again elsewhere if it was a real project.
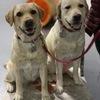
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Rana,
Compare your code that has properly-formatted markdown:
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
print(name)
print(number)
if number%3==0 and number%5==0:
print("is a fizzbuzz number")
elif number%3==0:
print("is a fizz number")
elif number%5==0:
print("is a buzz number")
else:
print("is neither a fizz nor a buzz number")
To code without:
name = input("Please enter your name: ") number = int(input("Please enter a number: ")) print(name) print(number) if number%3==0 and number%5==0: print("is a fizzbuzz number") elif number%3==0: print("is a fizz number") elif number%5==0: print("is a buzz number") else: print("is neither a fizz nor a buzz number")
Hopefully it is obvious that the version with markdown is much easier to read. However, there is more than just readability at stake. Consider the following example of broken code:
def broken_function(arg): for value in arg: if value: do_something() else: do_something_else()
It's actually impossible to detect the bug in the code without the markdown formatting.
Here's the same code with markdown:
def broken_function(arg):
for value in arg:
if value:
do_something()
else:
do_something_else()
Now it's possible to see the bug (the incorrect indentation of the else block).
Similarly, the syntax highlighting provided when you properly mark code samples with the language, helps you see other common errors at a glance. For example, compare how easy it is to see the error in the following code:
print('hello') print('there') print('this') print('is') print(printing) print('a') print('bunch') print('of') print('strings')
Now, see how easy it is to spot the error with syntax highlighting applied:
print('hello')
print('there')
print('this')
print('is')
print(printing)
print('a')
print('bunch')
print('of')
print('strings')
As for your other question, about which way of coding is the best. Obviously the most important thing is that the code actually works according to its specifications. Beyond that, everything is up for debate. Some people will argue that the shortest code is the best, either for performance reasons (all other things being equal, the shortest code will be quickest), or for reliability reasons (all other things being equal, every line of code has a chance of harbouring bugs, thus the shorter the code, the less room for bugs). Personally, my view is that the best code is the code that will be easiest for you, or your colleagues, to read if you come back to it in six months from now, having forgotten all the context that was in your mind when you wrote the code.
Hope that helps,
Cheers
Alex
Alex Koumparos
Python Development Techdegree Student 36,887 PointsAlex Koumparos
Python Development Techdegree Student 36,887 PointsPlease be sure to always format your code using the Markdown Cheatsheet (link below the text entry box) any time you post code. This is especially important with Python where whitespace is significant.