Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial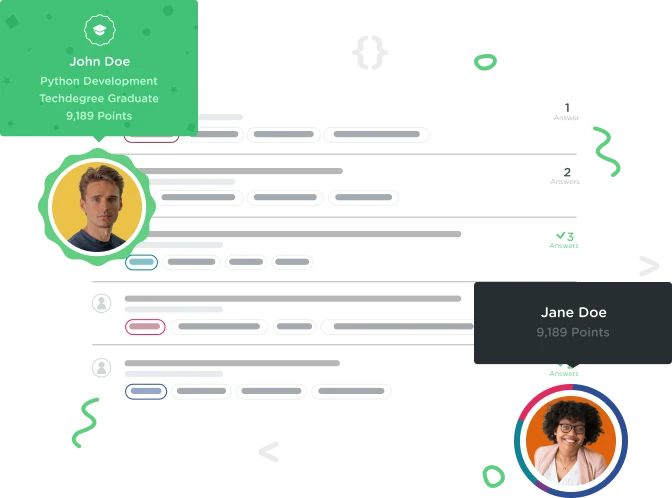
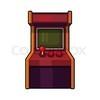
cyber voyager
8,859 PointsQuestion about my code
Hello, I started doing a mini project today and I made some buttons that if the key online in an object is true it styles it, something like one of the practices done in javascript basic's.
//variables
const ul = document.getElementById('ul');
const underscore = '_';
//Our user's info
let users = [{
firstName: 'Stavros', lastName: 'Pantelidis', online: false
}, {
firstName: 'Aris', lastName: 'Kokas', online: true
}];
function theCreator0(x) {
//The button
let makeBt = document.createElement('BUTTON');
if(users[x].online === true) {
makeBt.className = 'heIsOnline';
let iD = makeBt.id = underscore + x;
} else if(users[x].online === false) {
makeBt.className = 'heIsNotOnline';
let iD = makeBt.id = underscore + x;
}
makeBt.textContent = users[x].firstName;
//The button
//Make Ul and LI
let newLi = document.createElement('LI');
ul.append(newLi);
newLi.append(makeBt);
}
function theCreator1(x) {
//The button
let makeBt = document.createElement('BUTTON');
if(users[x].online === false) {
makeBt.className = 'heIsNotOnline';
let iD = makeBt.id = underscore + x;
} else if(users[x].online === true) {
makeBt.className = 'heIsOnline';
let iD = makeBt.id = underscore + x;
}
makeBt.textContent = users[x].firstName;
//The button
//Make Ul and LI
let newLi = document.createElement('LI');
ul.append(newLi);
newLi.append(makeBt);
}
for (let i = 0; i < users.length; i += 1) {
if (users[i].online === true) {
theCreator0(i);
} else if(users[i].online === false){
theCreator1(i);
}
}
<html>
<head>
</head>
<body>
<h1>Users Online</h1>
<ul id="ul"></ul>
</body>
</html>
* {
margin: 0;
padding: 0;
}
body {
background-color: black;
}
button {
position: absolute;
text-align: center;
width: 80px;
height: 25px;
border: none;
border-radius: 4px;
}
.heIsOnline {
left: 50%;
background-color: green;
color: blue;
}
.heIsNotOnline {
left: 50%;
background-color: red;
color: blue;
}
h1 {
position: absolute;
left: 46%;
color: yellow;
}
#_0 {
margin-top: 70px;
color: black;
}
#_1 {
margin-top: 80px;
color: black;
}
.neutral {
background-color: grey;
color: blue;
}
The thing is that the code is not 100% usable, what I mean buy that is that whenever we add a new object in our array(ourUser) it will not work and I want to use an instance of this code to make Ajax requests and practice a little bit. Most of the code is good but I would like some tips: how can I do it better? how the code can be more DRY? and how would I be able to make this code work whenever a user is added to our object? I know that the code is funny looking but that is my first legit mini-project. Thank you very much Stavros
1 Answer

Robin Whitting
5,964 PointsGood morning cyber voyager.
Firstly well done on putting together a working program using objects, and functions for your first time. I don't know what you mean about the program not working if you add another user. It worked as intended for me. You just don't have styles for the extra users.
I have re-factored your code to give you an example of what can be done to help conform to DRY principles. This is by no means the only way to accomplish this.
* {
margin: 0;
padding: 0;
}
body {
background-color: black;
}
button {
position: relative;
margin-top: 8px;
text-align: center;
width: 80px;
height: 25px;
border: none;
border-radius: 4px;
}
li:first-child {
margin-top:40px;
}
.heIsOnline {
background-color: green;
}
.heIsNotOnline {
background-color: red;
}
h1 {
position: absolute;
left: 50%;
transform: translateX(-50%);
color: yellow;
}
ul {
width: 80px;
height: 100vh;
}
body {
display: flex;
flex-direction: row;
justify-content: center;
}
.neutral {
background-color: grey;
color: blue;
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<h1>Users Online</h1>
<ul id="ul"></ul>
</body>
</html>
//variables
const ul = document.getElementById('ul');
const underscore = '_';
//Our user's info
let users = [
{
firstName: 'Stavros', lastName: 'Pantelidis', online: false
},
{
firstName: 'Aris', lastName: 'Kokas', online: true
},
{
firstName: 'Tom', lastName: 'Jones', online: true
}
];
function makeButton(logStatus, name) {
let makeBt = document.createElement('BUTTON');
makeBt.className = logStatus;
makeBt.textContent = name;
let newLi = document.createElement('LI');
ul.append(newLi);
newLi.append(makeBt);
}
function theCreator() {
for (let user of users) { // checks each user without index number
if(user.online === true) {
makeButton('heIsOnline', user.firstName);
}
else {
makeButton('heIsNotOnline', user.firstName);
}
}
}
if (users.length > 1) {
theCreator();
}
else{
console.error('There are no registered users');
}
Keep up the effort and fell free to ask questions if you don't understand anything.