Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial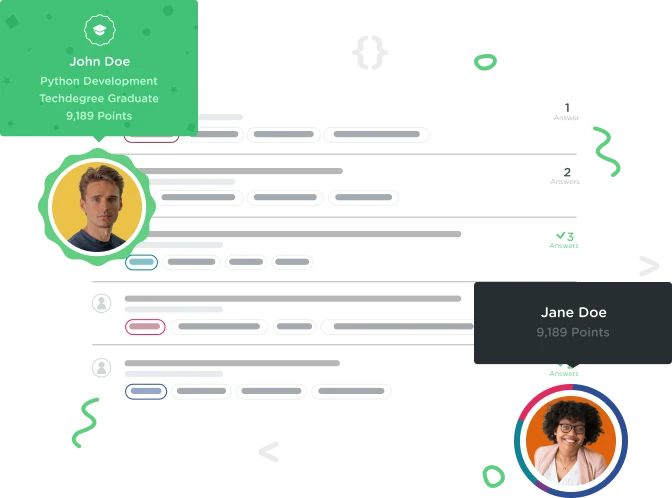

Sune Justesen
Courses Plus Student 3,838 PointsQuestion about my solution
I am doing this on an older but still decent laptop on Linux with Chrome. The code works fine, but some times when I press the button on the last question it lags for 5-10 seconds before it shows the finish screen, yet most of the time it is instant. Is there a problem with my code, or is my laptop just acting weird?
function Quiz() {
this.currentQuestionIndex = 0;
this.questions = [];
this.correctAnswers = 0;
this.addQuestion = (question) => {
this.questions.push(question);
};
this.guess = (response) => {
if (this.questions[this.currentQuestionIndex].answer === response.toLowerCase()) {
this.correctAnswers++;
}
this.currentQuestionIndex++;
return this.currentQuestionIndex === this.questions.length;
}
}
function Question(text, answer, choice0, choice1) {
this.text = text;
this.answer = answer;
this.choice0 = choice0;
this.choice1 = choice1;
}
const quizDiv = document.getElementById("quiz");
const questionText = document.getElementById("question");
const choice0 = document.getElementById("choice0");
const choice1 = document.getElementById("choice1");
const guessButton0 = document.getElementById("guess0");
const guessButton1 = document.getElementById("guess1");
const progressText = document.getElementById("progress");
quiz = new Quiz();
question0 = new Question("What is my first name?", "sune", "Sune", "Lars");
question1 = new Question("What is my last name?", "justesen", "Andersen", "Justesen");
quiz.addQuestion(question0);
quiz.addQuestion(question1);
const updateUI = () => {
const currentQuestion = quiz.questions[quiz.currentQuestionIndex]
questionText.innerHTML = currentQuestion.text;
choice0.innerHTML = currentQuestion.choice0;
choice1.innerHTML = currentQuestion.choice1;
let progressHTML = "Question ";
progressHTML += quiz.currentQuestionIndex + 1;
progressHTML += " of ";
progressHTML += quiz.questions.length;
progressText.innerHTML = progressHTML;
}
const finish = () => {
let finishHTML = "<h2>";
finishHTML += "Your score was ";
finishHTML += quiz.correctAnswers;
finishHTML += " out of ";
finishHTML += quiz.questions.length;
finishHTML += "</h2>";
quizDiv.innerHTML = finishHTML;
}
guessButton0.onclick = () => {
if (quiz.guess(choice0.textContent)) {
finish();
} else {
updateUI();
}
}
guessButton1.onclick = () => {
if (quiz.guess(choice1.textContent)) {
finish();
} else {
updateUI();
}
}
updateUI();

Sune Justesen
Courses Plus Student 3,838 PointsNo errors. I just tried again and can't replicate the problem. I think my laptop was just acting up. Thanks.
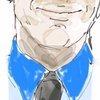
Abraham Juliot
47,353 PointsAlso, using .onclick to execute a function will overwrite previous calls to that function each time the element is clicked. You should instead use .addEventListener('click', () => { }, false). Generally, using .onclick is not an issue unless an element could be clicked again before the previous call to the function completes its exececution. This click event listener will not overwrite previous calls to the function. More info:

Sune Justesen
Courses Plus Student 3,838 PointsGood links, thanks. I don't overwrite the onclick property though. It's 2 different buttons, but I could make the code shorter with an eventlistener on the buttons' parent.
Reading your links I don't see anything about overwriting calls. How is that even possible?
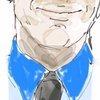
Abraham Juliot
47,353 Points.addEventListener takes a callback function which is executed asynchronously, meaning it does not block subsequent click events, while the .onlick method is a standard function which must complete itself before the next line of code. Thus, subsequent calls using .onclick will stop the current exection of the function in order to run it again.
This applies to all javascript functions. To prevent code from blocking, functions should be executed as callbacks. Here's an article on that subject: http://recurial.com/programming/understanding-callback-functions-in-javascript/
Abraham Juliot
47,353 PointsAbraham Juliot
47,353 PointsOpen up Chrome's dev tools. Do you see any console warnings or errors when the click event occors?