Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial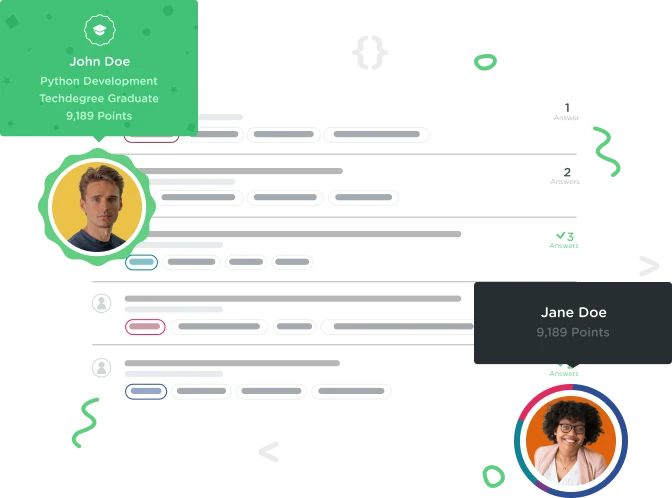
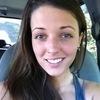
Amber Cyr
19,699 PointsQuestion about removing "concatScripts" from the build task
In the video, concatScripts was removed from the build task because it is a dependency of minifyScripts, so it will run regardless. Is this removal a requirement, or is it just for efficiency purposes of running the build task?
I only ask because I like being able to easily identify what tasks are being run within the build task, but since there is technically no need to identify the concatScripts, I feel it makes it harder to determine what the build task is actually running. Preferably, I would like to keep it in the dependencies list of the build task, unless someone can give me a good reason not to. Thanks in advanced!
'use strict';
var gulp = require('gulp');
var concat = require('gulp-concat');
var uglify = require('gulp-uglify');
var rename = require('gulp-rename');
var sass = require('gulp-sass');
var maps = require('gulp-sourcemaps');
gulp.task('concatScripts', function(){
return gulp.src([
'js/jquery',
'js/sticky/jquery.sticky.js',
'js/main.js'])
.pipe(maps.init())
.pipe(concat('app.js'))
.pipe(maps.write('./'))
.pipe(gulp.dest('js'));
});
gulp.task('minifyScripts', ['concatScripts'] function(){
return gulp.src('js/app.js')
.pipe(uglify())
.pipe(rename('app.min.js'))
.pipe(gulp.dest('js'));
});
gulp.task('compileSass', function(){
return gulp.src("scss/application.scss")
.pipe(maps.init())
.pipe(sass())
.pipe(maps.write('./'))
.pipe(gulp.dest('css'));
});
gulp.task('build',['minifyScripts', 'compileSass']); //concatScripts removed because it is a dependency of minifyScript but does it have to be?
gulp.task('default', ['build'] );
2 Answers
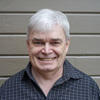
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,350 PointsI left the concatScripts task within the build task and it appears to work fine. So, yes, you can leave it in for helping track what is running.
gulp.task('build', ['concatScripts', 'minifyScripts', 'compileSass']);
$ gulp
[18:06:09] Using gulpfile ~\Gulp\treehouse-gulp-basics\gulpfile.js
[18:06:09] Starting 'concatScripts'...
[18:06:09] Starting 'compileSass'...
[18:06:09] Finished 'concatScripts' after 276 ms
[18:06:09] Starting 'minifyScripts'... //minifyScripts doesn't start until concatScripts is finished and it only runs once
[18:06:09] Finished 'compileSass' after 257 ms
[18:06:14] Finished 'minifyScripts' after 4.5 s
[18:06:14] Starting 'build'...
[18:06:14] Finished 'build' after 32 Ξs
[18:06:14] Starting 'default'...
[18:06:14] Finished 'default' after 16 Ξs
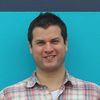
Sergio Alen
26,726 PointsHave you checked what shows in the terminal when you run it? I think it will just run 'concatScripts' twice, which wont cause issues. You could always add comments which is also a good idea to get used to.
Tata Gelashvili
3,076 PointsTata Gelashvili
3,076 PointsIt would be better if you can provide gulpfile.js code here :) After it I can answer your question.