Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial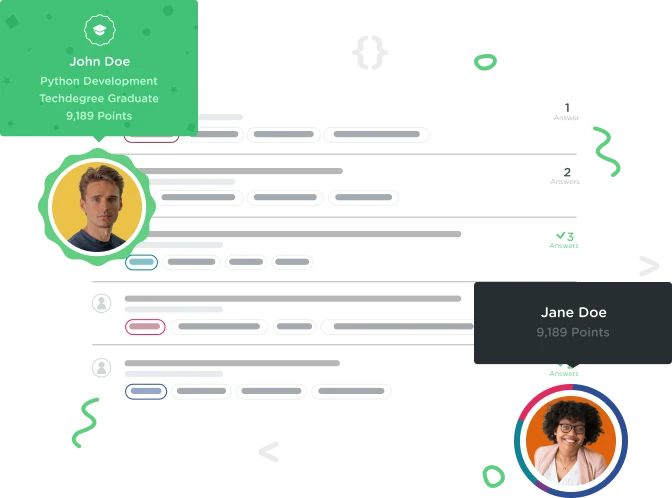
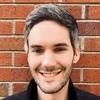
Jesse Vorvick
6,047 PointsQuestion about scope
In the solution, the instructor did the following:
function printList( results ) {
var listHTML = '<ol>';
for (i = 0; i < results.length; i += 1) {
listHTML += '<li>' + results[i] + '</li>';
}
listHTML += '</ol>';
return (listHTML);
}
Why 'return'? Why not:
function printList( results ) {
var listHTML = '<ol>';
for (i = 0; i < results.length; i += 1) {
listHTML += '<li>' + results[i] + '</li>';
}
listHTML += '</ol>';
printList (correct);
}
It should be noted that in the second example, nothing was printed to the webpage, and in the console 'listHTML' returns as "This variable is not defined." I'm guessing this has to do with scope? But this was how it was done in the video in the teacher's notes we were told to reference, so I don't understand why or the importance of 'return'.
3 Answers
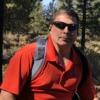
Mark Kastelic
8,147 PointsJesse,
The code below works. Is this what you are trying to do?
var questions = [
['How many states are in the United States?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0;
var question;
var answer;
var response;
var correct = [];
var wrong = [];
function print(message) {
document.write(message);
}
function printList(results) {
var listHTML = '<ol>';
for (i = 0; i < results.length; i += 1) {
listHTML += '<li>' + results[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
response = parseInt(response);
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
print("You got " + correctAnswers + " question(s) right.");
print('<h2>You got these questions correct:</h2>');
printList(correct);
print('<h2>You got these questions wrong:</h2>');
printList(wrong);
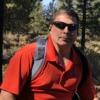
Mark Kastelic
8,147 PointsHi Jesse,
I believe you could use a "print" instead of "return." But, in your code I think you did a typo and used "printList" instead (which just calls the function again).
The function is most generically used when it provides a return, since an html string can be built up from multiple calls to the function, along with other concatenations along the way before finally being printed out.
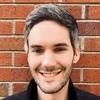
Jesse Vorvick
6,047 PointsThank you! I can't believe I missed that. But even when changing it to "print(listHTML)" it still doesn't work, even with "printList(correct)" placed below and outside the function. It makes me wonder if having both print(html) and printList(correct) is the problem. one print will overwrite the other or something.
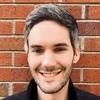
Jesse Vorvick
6,047 PointsHey, thanks for your effort in helping me! At this point I think it is too late to remember what I did when, and why whatever worked and whatever didn't. However, I'm glad to know from your reply that calling the print function more than once doesn't cause it to overwrite itself; that was the main thing I was wondering.