Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial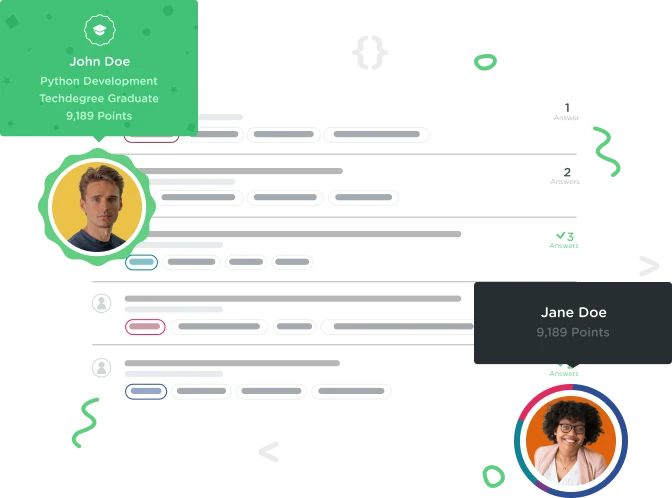
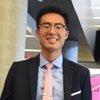
Paul Je
4,435 PointsQuestion about Syntax
What part of this would be wrong? Based on the question I tried to add the function itself as a parameter of mathOperation, but it seemed Xcode didn't like it. I instead added the parameters of the previous function as the parameters of the new one and it seemed like Xcode doesn't have any problems with it. As well, the error message was informing me that I needed to make the return type Int, which I already have done. I'm confused about when I should include an open brackets and when not to, and I have a sneaking suspicion I should have included it after the Int type was declared, but I don't understand what that would imply if I did so I am stuck. Thanks for any help..
/**
For this code challenge, letβs define a math operation as a function that
carries out some work on two integers and returns an integer as well. An
example is the function below, `differenceBetweenNumbers`, which takes two
integers and calculates the difference between the numbers. After calculating,
it returns the difference.
*/
func differenceBetweenNumbers(a: Int, b: Int) -> (Int) {
return a - b
}
// Enter your code below
func mathOperation(a: Int, b: Int) -> (Int) {
return mathOperation(3, 1)
}
1 Answer
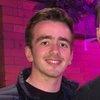
Stephen McMillan
iOS Development with Swift Techdegree Graduate 33,994 PointsWhat you have added above is a function that accepts two Ints, a and b then returns an Int.
The challenge says to create a function that:
- Accepts another function that has the same 'signature' as the differenceBetweenNumbers function.
- Accepts two integers on which to perform the 'mathOperation'.
- Returns an Integer.
As mentioned, the function has to accept a function as a parameter type. Like it says in the Swift docs you do this simply by writing the following as one of the parameters to your 'mathOperation' function.
operation: (Int, Int) -> Int
Then, all you do is say the function should take two other Ints that you want to perform the passed in operation on and return an Int as well. So that would form a function signature like this.
func mathOperation(operation: (Int, Int) -> Int, a: Int, b: Int) -> Int {}
Finally, you just call the operation passed in on a and b and return the value inside the function you just wrote called mathOperation.
return operation(a, b)
Hope this helped! :)