Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial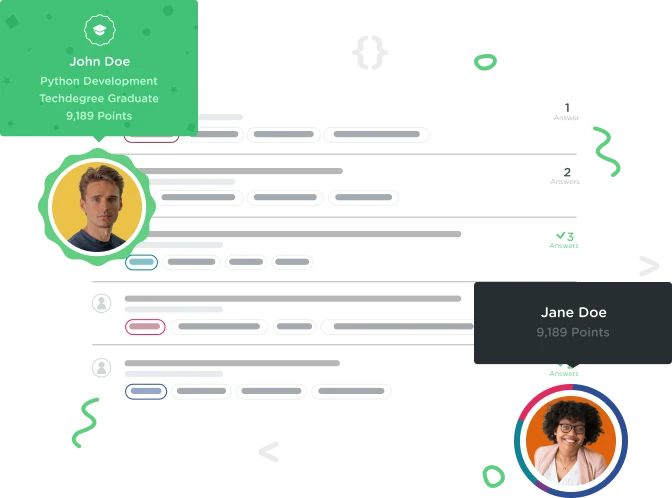

Hussein Amr
2,461 PointsQuestion about the opposite guessing game
so here's the thing, as you can see from my code i'm trying to find a way to make the "too low" thing work but i can't .. any ideas on how to get more creative about it?
import random
#user chooses the secret number
try:
secret_num = int(input("Enter your secret number that you'll want me to guess: "))
except ValueError:
print("{} is not a number ".format(secret_num))
#Here we rerun the game again
else:
def game():
guess = random.randint(1 , 10)
new_guess = random.randint(1, 10)
higher_guess = new_guess > guess
print(guess)
#computer tries to guess it
#return if hit or miss
while True:
my_reply = input("> ")
if my_reply == ("correct"):
print("nice")
break
elif my_reply == ("too low"):
higher_guess
game()
game()

Hussein Amr
2,461 PointsSteven Parker I'm really sorry it's kind of a habit i didn't notice. anyway i updated the script, and chance of helping now ?
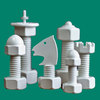
Steven Parker
231,269 PointsSome habits might be good to break!
1 Answer
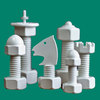
Steven Parker
231,269 PointsHere's a few hints.
In your code, "higher_guess" is a boolean that indicates of a second random number was larger than the first one. But both numbers are being picked from the same range (1-10) so it's not really anything useful. And using it on a line by itself doesn't do anything, like you have after the user enters "too low". And then you call game which starts everything over again.
What you'd probably want to do instead is expand the loop so that it includes the printing of the current guess. Then, if the player says "too low" pick a new random number but instead of 1 to 10, pick from guess + 1 to 10. Then put that back into guess and let the loop repeat instead of starting over. That way, when the user says "too low" your next guess will always be more than the one that was too low.
To make the program even smarter, you might keep track of both "too low" and "too high" responses, and pick new random numbers in between.
You might take a look at other questions by people working on the same thing for ideas on this and other enhancements.

Hussein Amr
2,461 PointsTried it but still not working :( giving make 10 everytime in the second try
import random
#user chooses the secret number
try:
secret_num = int(input("Enter your secret number that you'll want me to guess: "))
except ValueError:
print("{} is not a number ".format(secret_num))
#Here we rerun the game again
else:
def game():
guess = random.randint(1 , 10)
print(guess)
#computer tries to guess it
#return if hit or miss
while True:
my_reply = input("> ")
if my_reply == ("correct"):
print("nice")
break
elif my_reply == ("too low"):
print(random.randint(guess, 10))
game()
game() ```
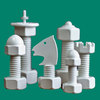
Steven Parker
231,269 PointsYou haven't finished implementing my suggestions yet. I'll break them out into a checklist:
- expand the loop so that it includes the printing of the current guess
that means make the original print part of the loop instead of adding another one - if the player says "too low" pick a new random number from guess + 1 to 10
- put that back into guess
- let the loop repeat instead of starting over
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou might want to revise your code to use different language in your messages before a mod or staff member sees it!
And I might give you some hints myself after you change it.