Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial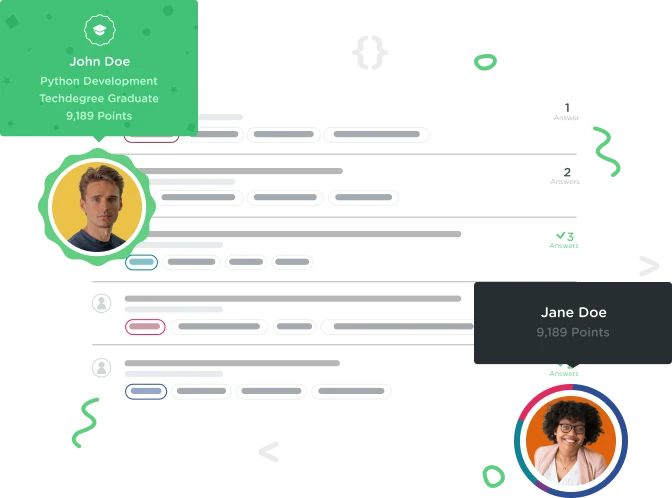
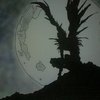
Lucas Santos
19,315 PointsQuestion about this Quiz
So I passed this quiz but did not fully understand the code. What I did not understand is how we accessed the getAuthor method from the BlogPost class if we never instantiated that class in the Blog class to begin with. Here is my code:
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public Set<String> getAllAuthors() {
Set <String> authors = new TreeSet<String>();
for (BlogPost author : mPosts) {
authors.add(author.getAuthor());
}
return authors;
}
public List<BlogPost> getPosts() {
return mPosts;
}
}
So the part with
for (BlogPost author : mPosts) {
authors.add(author.getAuthor());
}
How is mPosts accessing BlogPosts method of getAuthor without it instantiating the BlogPost class?
1 Answer
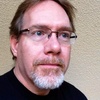
Chris Freeman
Treehouse Moderator 68,423 PointsThe BlogPost
class is accessible because it is part of the same com.example
package. BlogPost
is Instantiated in the Blog constructor when it assigns the lists of BlogPosts (via posts
) to the member field `mPosts.
The code snippet is a bit confusing by using the parameter name "author". It would read better if it was called "post":
for (BlogPost post : mPosts) {
authors.add(post.getAuthor());
}
Now it reads "for each 'post' in the mPosts
list of BlogPosts
, get the author and add it to Set
of author names strings.
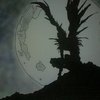
Lucas Santos
19,315 PointsStill don't really understand, do you mean it gets instantiated when we create a new Blog object and pass in a List of BlogPosts there for giving mPosts a value?
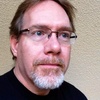
Chris Freeman
Treehouse Moderator 68,423 PointsCorrect. The challenge instructions say "[Blog] is initialized with a list of BlogPost objects." which implies there is other code somewhere that executed the equivalent of
Blog someBlog = new Blog(listOfBlogPosts);
This "list of blog posts" would then get assigned to the the mPosts
in Blog
. Each of the posts in the array would have the .getAuthor()
method.
There is no obvious mechanism in the challenge code that shows how BlogPosts
get added to the Blog
list of BlogPosts
other than in the Blog constructor. It would have been clearer if there were an addPost
method in Blog
Tagging @Craig Dennis for comment.
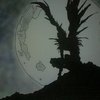
Lucas Santos
19,315 PointsI see so then we would do something like
someBlog.getAllAuthors ();
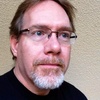
Chris Freeman
Treehouse Moderator 68,423 PointsExactly! Additionally, other methods of Blog
could reference it locally as:
// From within Blog
Set<String> allAuthors = getAllAuthors();
// From some other code
Set<String> allAuthors = someBlog.getAllAuthors();
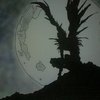
Lucas Santos
19,315 PointsI see ok think I got it but just one last concern. You said "The BlogPost class is accessible because it is part of the same com.example package."
What do you mean by that exactly? and if it wasn't in the same package we would need to instantiate it?
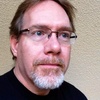
Chris Freeman
Treehouse Moderator 68,423 PointsWhat I meant by "The BlogPost class is accessible because it is part of the same com.example package" is that the package
statement in Blog.java declares the Blog
class to be in the package "com.example". This is the same package declared in BlogPost.java for the BlogPost
class. By being in the same package, the public declarations in both java files are visible to each other. If you remove the package
statement from either java file, references to BlogPost
inside of Blog.java would not be resolvable.
In other words, When compiling a java file, all of the type definitions must be in either
- the same file
- a file in the same package
- a file listed in an import statement from another package
- or built-in types
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI see, ok got it thanks a ton Chris.