Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial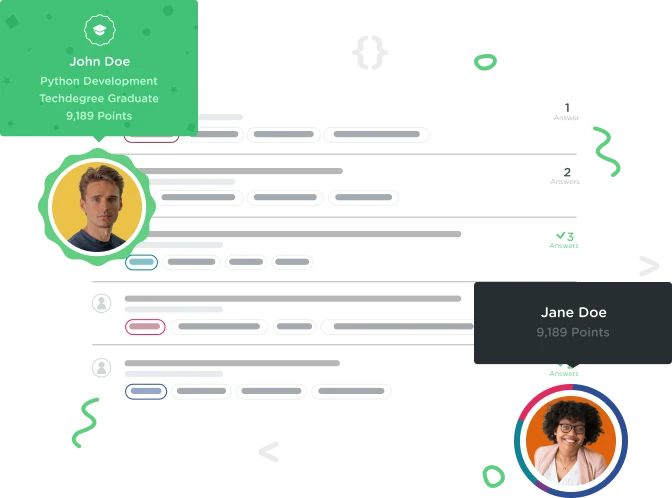
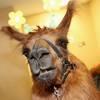
A X
12,842 PointsQuestion about Using Colon vs. Equals Sign in Challenge
From the challenge here: https://teamtreehouse.com/library/javascript-loops-arrays-and-objects/tracking-data-using-objects/add-properties-to-an-object
I originally put in for the solution (out of habit) to this challenge (which I know is wrong but not why):
var paris = {
population = 2.211e6,
latitude = '48.8567 N',
longitude = '2.3508 E'
};
So I get it that we're assigning values to the properties of our object, but up until this point we've used the equals sign ( = ) to assign something to a variable, so why are we switching now to the colon ( : ) ? Is this just a case of "we just use a colon here because that's just what we use in objects?" ?
1 Answer

Aaron Martone
3,290 PointsYou're using "Object Literal Notation" when creating an object in that manner. The proper syntax for it is keyName: keyValue
. This is different than Assignment
that uses the =
operator. In essence, yes, you are doing the same thing (assigning a value to an identifier), but literal syntaxes are known as shorthand. If you wish to use the =
for all your assignments, you'll want to change to Dot Notation Syntax
, like:
var myNewObject = {};
myNewObject.property1 = value1;
myNewObject.property2 = value2;
/* etc... */
As you can see, it would be much slower to do this, so the Object Literal syntax is highly favored.
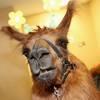
A X
12,842 Points@Aaronmartone I appreciate you explaining the difference between the object literal notation and the dot notation & the example in dot notation. With your example I can see why the literal notation is favored. The dot vs. object literal concept wasn't gone over in Dave's video introducing the subject. Thanks!
Aaron Martone
3,290 PointsAaron Martone
3,290 PointsYou're welcome. To expand as well, though not preferred, you can name keys on an object
'look ma spaces'
. In doing so, it would be impossible to use dot notation syntax to reference that property, key:myObject.look ma spaces; // The spaces in the property key name break the interpretor's ability to resolve it.
That's why we also have
Associative Array Notation
. Simply put, you put[]
next to the object and specify the name of the property in a literal string, like this:myObject['look ma spaces']; // This works and will return the value on the key.
That said, I consider it bad form to use spaces in key properties. If you need spacing, use an underscore (
_
).