Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial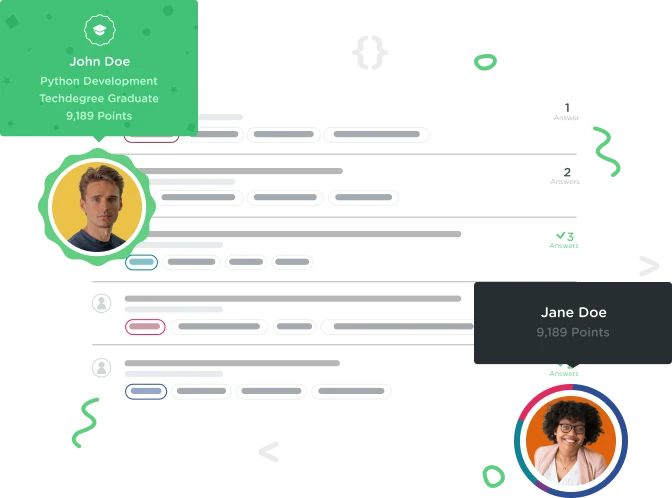
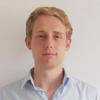
jornemoonen
6,616 PointsQuestion counter doesn't seem to work + feedback on my code?
- I'am trying to get the question counter to work in this challenge but it seems it doesn't count down like it should?
- Overall feedback on my code to improve is always welcome. Thanks You in advance!
/* CHALLENGE INSTRUCTIONS
1. Ask at least five questions
2. Keep track of the number of questions the user answered correctly
3. Provide a final message after the quiz letting the user know the number of questions he or she got right.
4. Rank the player. If the player answered all five correctly, give that player the gold crown: 3-4 is a silver crown; 1-2 correct answers is a bronze crown and 0 correct is no crown at all.
*/
/* Challenge Question + Answer
1. Where stands WWW for? World Wide Web
2. What is the color black in CSS? #000
3. What is 101 in binary code? 5
4. The brain of a computer is? CPU
5. Mega Byte is equel to? 1024 Kilo Bytes
*/
// Start Quiz
console.log("Start Quiz");
// Score
var answer = 0;
// Counter questions remaining
var questions = 5;
var questionsLeft = "[" + questions + " " + "questions left]";
// Question 1
var questionOne = prompt("Where stands WWW for?" + questionsLeft);
if (questionOne.toUpperCase() === "World Wide Web") {
alert("You're correct!");
answer += 1;
questions -= 1;
questionsLeft = '[' + questions + ' questions left]';
} else {
alert('Sorry, this is incorrect.');
}
// Question 2
var questionTwo = prompt("What is the color black in CSS?" + questionsLeft);
if (questionTwo.toUpperCase() === "#000") {
alert("You're correct!");
answer += 1;
questions -= 1;
questionsLeft = '[' + questions + ' questions left]';
} else {
alert('Sorry, this is incorrect.');
}
// Question 3
var questionThree = prompt("What is 101 in binary code?" + questionsLeft);
if (parseInt(questionThree) === 5) {
alert("You're correct!");
answer += 1;
questions -= 1;
questionsLeft = '[' + questions + ' questions left]';
} else {
alert('Sorry, this is incorrect.');
}
// Question 4
var questionFour = prompt("The brain of a computer is?" + questionsLeft);
if (questionFour.toUpperCase() === "CPU") {
alert("You're correct!");
answer += 1;
questions -= 1;
questionsLeft = '[' + questions + ' questions left]';
} else {
alert('Sorry, this is incorrect.');
}
// Question 5
var questionFive = prompt("Mega Byte is equel to?" + questionsLeft);
if ( questionFive.toUpperCase() === "1024 Kilo Bytes") {
alert("You're correct!");
answer += 1;
questions -= 1;
questionsLeft = '[' + questions + ' questions left]';
} else {
alert('Sorry, this is incorrect.');
}
// Ranking the player based on his score
if (answer === 5) {
document.write("<p>You have won a GOLDEN CROWN!</p>");
} else if (answer <= 4 && answer >= 3) {
document.write("<p>You have won a SILVER CROWN!</p>");
} else if (answer <=2 && answer >= 1){
document.write("<p>You have won a Bronze Crown!</p>");
} else if (answer === 0) {
document.write("<p>Sorry, You didn't win a Crown.</p>");
}
// Displaying the final score
var finalResult = alert("Congratulations you answered " + answer + " questions correctly!");
// End Quiz
console.log("End Quiz");
2 Answers

Joseph Frazer
5,404 PointsWell, didn't really get what you are asking because the counter did work. But, make sure you do:
if (questionOne.toUpperCase() === "WORLD WIDE WEB") {
It is uppercase.
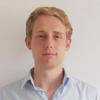
jornemoonen
6,616 PointsIf you test the code above you will see that "questions left" always stay's on "5 questions left" and never goes to "4 questions left" and so on. "Thank you for the tip about the upper case letters
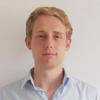
jornemoonen
6,616 Points@Martin Balon I wasn't sure if I had to do it also when the user fails but now I know I should. Now that you mention about the upper case in the condition, it makes sense. Thank you
Martin Balon
43,433 PointsMartin Balon
43,433 PointsHi Jorne, your questions counter doesn't work when user fails at answering question - include 'questions--;' to each else block so even if user fails - the questions left is up to date. Also as Joseph pointed out you are comparing user's answers which are all in upper case meanwhile the condition in if block is in lower case - so user will always fail....