Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial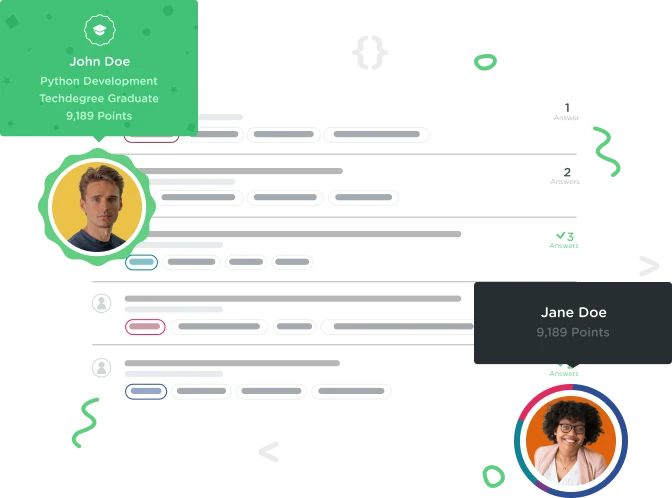

Boj H
5,021 PointsQuestion of Challenge task 1 of 1 at Stage 5 Functions
Challenge task 1 of 1
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
My code has problem to check ['string 23'] as an array, please help me out. Thanks.
<script>
function arrayCounter (a)
{
if (typeof a==='undefined') return 0;
if (!isNaN(a)) return 0; /* number */
var str_a = a.toString();
var aa = str_a.split(',');
console.log(aa);
console.log(aa.length);
if (aa.length>1) return a.length;
else return 0;
}
console.log(arrayCounter('string 23'));
console.log(arrayCounter());
console.log(arrayCounter(2));
console.log(arrayCounter('23'));
console.log(arrayCounter(['1',2,3]));
console.log(arrayCounter['string 23']);
</script>
5 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Boj,
The way that you're checking if the type is 'undefined' is the same thing you want to do for 'string' and 'number'. You want to use the typeof
operator on each one and return 0 if it's any of them. If not, then you can return the length of the array.
function arrayCounter (a)
{
if (typeof a === 'undefined') {
return 0;
}
if (typeof a === 'string') {
return 0;
}
if (typeof a === 'number') {
return 0;
}
return a.length;
}
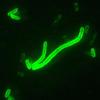
Binary Soul
4,592 Pointsif (!isNaN(a)) return 0;
Means if 'a' is-not-not-a-number as far as i can see.
have you tried:
if (isNaN(a)) return 0;
?
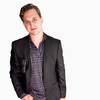
Cody Thompson
Courses Plus Student 8,430 PointsThe way I solved the challenge was as following:
function arrayCounter(a){
if(typeof a === 'undefined' || typeof a === 'number' || typeof a === 'string' ){
return 0;
} else {
return a.length;
}
}
I think you were simply over-thinking the question haha.
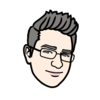
pauloliva
7,889 PointsI did it that way and it gave me errors. :(
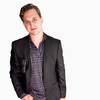
Cody Thompson
Courses Plus Student 8,430 PointsIt shouldn't give you an error if you did it correctly. Read the way you wrote out your initial question;
"return the length of an array passed in OR 0 if a 'string', 'number' OR 'undefined' value is passed in."
"||" = logical OR operator.
Writing it out Jason's way isn't incorrect but in my mind its a bit more semantically correct and less repetitive to use the operator.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_Operators
Take a quick look at that page to read about it.

Jason Anello
Courses Plus Student 94,610 PointsHi Cody,
The only reason I didn't use logical OR is because I don't think it was covered up to that point in the course. I try to give solutions that are based on course material and optionally show better solutions.
It does remove the repetition but it's still not the ideal solution.

Arthur Morgan
5,919 PointsWhy does this not work? Maybe I've got the syntax wrong, but what I'm getting at is why the concept doesn't work. Instead of undefined or number or string why not just go for 'not an array'?
function arrayCounter(a){
if(typeof a !== 'array'){
return 0;
} else {
return a.length;
}
}

Jason Anello
Courses Plus Student 94,610 PointsHi Arthur,
This doesn't work because the typeof
operator doesn't return the string 'array'
See this mdn page on the typeof operator to see the list of possible strings that it can evaluate to: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/typeof
You can see the solution I posted above using Array.isArray()
which is more along the lines of what you are trying to do here.

Tracey Dolby
5,638 PointsVery confused! Can anyone explain to me why the code needs to be done this way: function arrayCounter (a) { if (Array.isArray(a)) { // It's an array, return the length return a.length; }
// It wasn't an array, return 0
return 0;
or the way that Cody wrote? I don't understand using // (thought this removed phrases from the code) or || (which according to the explanation is the or value, but I don't remember going over this in any videos) I've read through the forum, and my notes from the videos but I still don't remember seeing any code written these ways. An explanation would help me better understand this challenge.
Thanks, Tracey
pauloliva
7,889 Pointspauloliva
7,889 PointsIs there any way to check that an array it's in fact an array and not an object?
Like when we use typeof(array) it will return as 'object'.
Even when using return array.isArray it will also return the value as an object.
Is there a function that will return 'array' instead of 'object'?
I have checked some answers on stack overflow but they are very confusing.
Like this one:
isArr = Object.prototype.toString.call(data) == '[object Array]';
From where is the 'data' value coming from?
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi pauloliva ,
Here's the MDN page for
isArray
: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/isArrayThe syntax is
Array.isArray(obj)
. you're callingisArray()
on theArray
object. Note the capital 'A'You pass in the thing you're checking and it will return a
true
orfalse
value depending on whether it was an array or not.A solution using isArray could look like this:
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI forgot to answer your question about the code you found.
There's a polyfill on the link I gave you:
This contains code similar to what you found on stackoverflow.
If you drop that code in first then you will be able to use isArray even if it's not natively available on older browsers.