Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial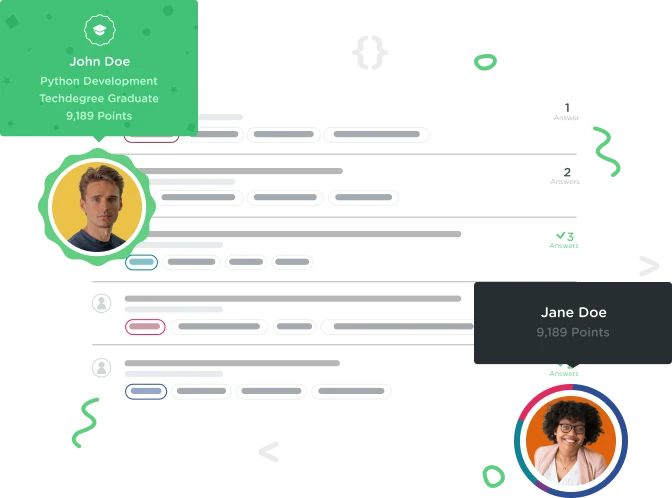

Robert Kendall
1,287 PointsQuestion on getter
I think I'm on the right track, but I may be way off.
I added a parameter to the spaceship class in spaceship.java, then tried to pull the same parameter out of Landing activity.java.
I couldn't understand the syntax errors.
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends Activity {
public Button mThrustButton;
public TextView mTypeLabel;
public EditText mPassengersField;
public Spaceship mSpaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
mThrustButton = (Button)findViewById(R.id.thrustButton);
mTypeLabel = (TextView)findViewById(R.id.typeTextView);
mPassengersField = (EditText)findViewById(R.id.passengersEditText);
// Add your code here!
mSpaceship = new Spaceship("FIREFLY",numPassengers);
mTypeLabel.setText(mSpaceship.getType());
mPassengersField.setText(mSpaceship.getnumPassengers().toString());
}
}
public class Spaceship {
private String mType;
private int mNumPassengers = 0;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public int getNumPassengers() {
return mNumPassengers;
}
public void setNumPassengers(int numPassengers) {
mNumPassengers = numPassengers;
}
public Spaceship() {
mType = "SHUTTLE";
}
public Spaceship(String type, int NumPassengers) {
mType = type;
mNumPassengers = numPassengers;
}
}
2 Answers
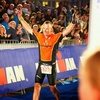
Steve Hunter
57,712 PointsHi Robert,
There's not much to comment on with your code - you're pretty much there!
Here's my solution:
mSpaceship = new Spaceship("FIREFLY");
mTypeLabel.setText(mSpaceship.getType());
mPassengersField.setText(mSpaceship.getNumPassengers() + "");
You don't need to mess with numPassengers
in the constructor; that just takes a string. Your mTypeLabel
is spot on and the mPassengersField``` piece looks OK - I need to try your code to see if that's not what the compiler is expecting. I just used a 'moosh' for a quick conversion to a string.
Hope that helps!
Steve.

Ajith vishwanath
Courses Plus Student 295 PointsI am having errors....I got struck in this place..pls help me
https://secure.helpscout.net/file/25179109/ee5a821f3b27e970825bc18df36d70a134dbc71f/Capture.png
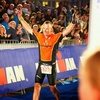
Steve Hunter
57,712 PointsHi Ajith vishwanath ,
You have a class called MyProject
that extends the Color
class.
You need to extend an Activity
of some sort, else th methods you're trying to use, such as super.onCreate()
and findViewById()
aren't methods of the class.
If you change your first line to extend a different class - the original project used ActionBarActivity
but that may be deprecated now - then this should work. You don't want to extend Color
. Try it with ActionBarActivity
or just Activity
and see what happens.
Hope that helps a little.
Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsTwo issues in your last line. First, there's a slight typo in the method name,
getnumPassengers()
- that needs a capital 'N'. And it isn't liking the use oftoString()
.I think that's because
toString()
is a class method ofInteger
. So you would use that likeInteger.toString(i)
.Putting that in the code here might look like:
mPassengersField.setText(Integer.toString(mSpaceship.getNumPassengers()));
I hope that helps!!
Steve.