Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial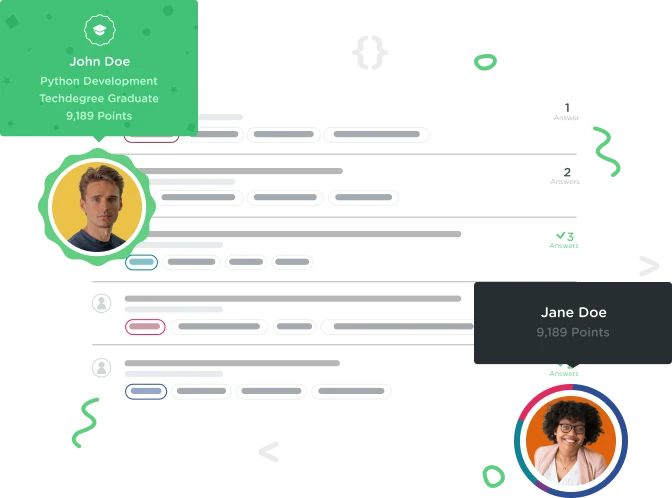

Joel K
3,845 PointsQuestion on Objects Section of Intro to Programming
I have the following code...
var me = {
first_name: "Adam",
last_name: "Smith",
"Employee Number": 1
}
console.log(me.first_name);
console.log(me.last_name);
console.log(me["Employee Number"]);
var key = "first_name"
console.log(me[key]);
http://jsbin.com/opIDejoZ/1/edit
So i get the whole concept of the first block. The object me has properties and i can console.log those to display the key values. I'm just wondering about the last part with the var key="first name". How does the JS know that first_name is looking for the first key/value pair in the me object. Why does it not see "first_name" as a string and therefore perhaps different than the key first_name in the object above it?
I hope i was clear. This is probably a simple concept i'm missing.
Thanks.
4 Answers

eirikvaa
18,015 PointsDoing
console.log(me.first_name);
is exactly the same as doing
console.log(me["first_name"]);
The latter is convenient when you have spaces in your key. You couldn't access the Employee Number like below.
console.log(me."Employee Number");

James Barnett
39,199 PointsWhat's going on there is JS is looking at the string first_name
and then checking the object me
and seeing if there is a key with the name first_name
and returning it's value adam
.
I hope I explained that clearly enough.
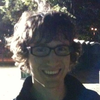
Ben Rubin
Courses Plus Student 14,658 PointsIt actually does see first_name
as a string. key
is a variable that you are setting to the string "first_name". That's what the double quotes mean. The line me[key]
tells JS to go to the me
object and get the property named first_name
(since key
contains the string "first_name").
You can do a test to see that. Try these two lines.
console.log(me["first_name"]);
console.log(me[key]);
You'll see that those both give the same result. Then try this line.
console.log(me[first_name]);
You see that that produces an error since first_name
is neither a variable nor a string value.

Joel K
3,845 PointsThanks everyone. Strange concept to wrap my head around but think i understand it.
Adam Sackfield
Courses Plus Student 19,663 PointsAdam Sackfield
Courses Plus Student 19,663 PointsIn the final console.log you are passing it the variable object "me"