Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial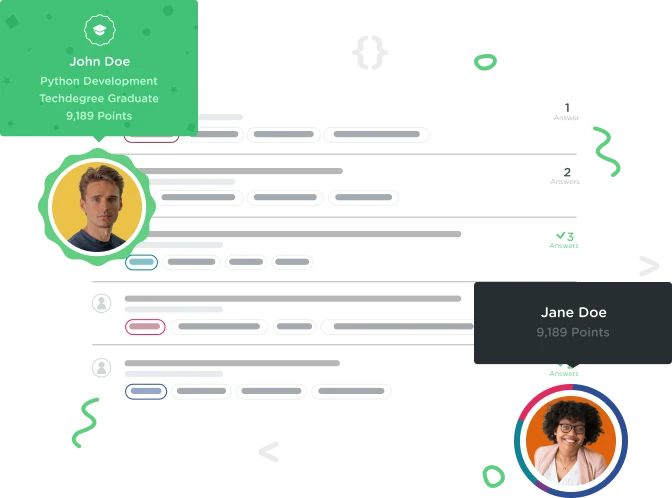
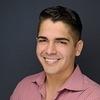
ian izaguirre
3,220 PointsQuestion on Scope in For loops and Array literals ?
First Question on Scope:
// Student records
var students = [
{
name: 'Ian',
track: 'iOS',
achievements: 5,
points: 120
},
{
name: 'Juan',
track: 'Design',
achievements: 32,
points: 450
}
];
function print(message) {
document.write(message);
}
var name;
var j = students.length;
for ( var i = 0; i < j; i+=1 ) {
name = students[i].name;
print( name );
}
The Above Code works ... BUT when I change declaring the variable name
outside of the For Loop :
// Student records
var students = [
{
name: 'Ian',
track: 'iOS',
achievements: 5,
points: 120
},
{
name: 'Juan',
track: 'Design',
achievements: 32,
points: 450
}
];
function print(message) {
document.write(message);
}
var name = students[i].name;
var j = students.length;
for ( var i = 0; i < j; i+=1 ) {
print( name );
}
I get undefined? I do not understand how this gives an error if only functions have local scope?
2 Answers
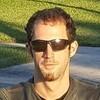
Joshua Kaufman
19,193 PointsYou might need to play around with it a bit to get a stronger feel for it.
"i" MUST be an integer to pull values out of an array, since arrays have index numbers to be identified (starting at 0).
When I gave you that code snippet, we set i = 0. Since i = 0 before running students[i].name, it looks for students[0].name, which is the name VALUE inside of the FIRST object. If you did students[1].name, it would pull "Juan" instead because the second object (at index 1) has a name VALUE of "Juan".
When you fail to initial "i", or assign it to a string, it CAN'T find an index inside of the students array to go off of, and won't give you anything useful: students[''].name doesn't make sense to a computer in JavaScript.
//name = students[''].name doesn't work because '' is not an integer
Likewise, when you don't initialize the value for "i", it stays undefined. students[undefined].name means nothing to the computer, and can't be found at all.
///name = students[undefined].name doesn't work because it doesn't make logical sense, and is also not an integer
We use the for loop to get all the values because it automatically sets AND increments "i". When "i" is the loop counter, it is not only a number but also the number you NEED.
This is the first iteration
for(var i = 0; i < students.length; i++) //IMPORTANT! students.length = 2
{
//runs at i = 0 because 0 < 2;
name = students[i].name; // gives "name" the value of students[0].name, which is "Ian"
print( name ); //runs the print function
}
This is the second interation
for(var i = 0 i < students.length; i++) //WHEN THIS RUNS AGAIN, i = 1
{
name = students[i].name //gives "name" the value of students[1].name, which is "Juan"
print(name); //runs the print function
}
Basically, for loops are sick for writing stuff out of arrays AND objects, you need integers to do it in JavaScript, and anything else won't work.
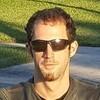
Joshua Kaufman
19,193 PointsThe "i" variable gets thrown out of memory right after the loop ends. Outside of it, "i" isn't recognized as a variable. Since code reads top to bottom, it will try to find the index "i" for students.name, but "i" doesn't exist! "i" ONLY has meaning within the loop.
You COULD assign var i = 0 right above the var name = students[i].name; line...
//like this
var i = 0
name = students[i].name;
//name equal "Ian" when you do this, but we use for loops to go through ALL of the entries.
I wouldn't recommend it though, since you won't get all of the values from your array of objects.
Does that make more sense? If not, I'll try to clarify more.
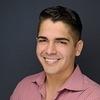
ian izaguirre
3,220 PointsThank you, I understand now my mistake. Also by doing it in a function it works:
var i;
function startProgram() {
var name = students[i].name;
var track = students[i].track;
print( name + track );
}
var j = students.length;
for ( i = 0; i < j; i+=1 ) {
startProgram();
}
ian izaguirre
3,220 Pointsian izaguirre
3,220 PointsAwesome, thank you for taking the time to give such an in depth answer.