Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial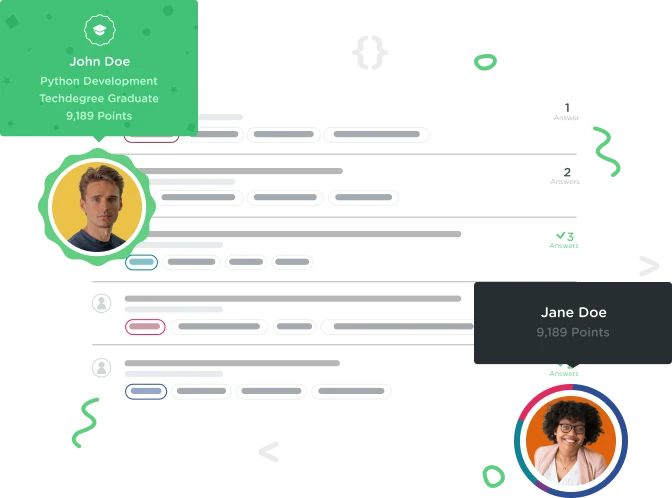

Marvin Benno
7,524 PointsQuestion regarding my use of the print function.
In my code I have set up the function print like Dave did in the vide. I'm using it to print out three different strings. html, htmlRight and htmlWrong. It seems like in my code that I can't use the same print function for all three prints. If I call the print function three times using different variables inside the parentheses it only prints the last one in the code. Can someone explain if I'm doing something wrong and how I can make the print function work like i want it to work and print three times with different variables inside. Hope this makes sence, appriciate some help :D
<!DOCTYPE HTML>
<html>
<head>
<meta charset="utf-8">
<title>Shaba</title>
</head>
<body>
<h1>
This is my Javascript site.
</h1>
<div id="output">
</div>
<script src="javascript.js" type="text/javascript"></script>
</body>
</html>
var arr = [
["Hur lång är Marvin Benno? ", 2],
["Är Putin och McGregor vänner? ", 2],
["Hur många dagar finns på ett år? ", 2],
["Vart fiskar Marvin gädda? ", 2],
["1?", 2]
];
var questionsAsked = 0;
var wrong = 0;
var right = 0;
var answer;
var html;
var htmlRight = "<div> <h1> You got these questions right </h1> <ol>";
var htmlWrong = "<div> <h1> You got these questions wrong </h1> <ol>";
var questionsRight = [];
var questionsWrong = [];
function print(message){
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for (var i = 0; i < arr.length; i++) {
answer = prompt(arr[i][0]);
questionsAsked += 1;
if (parseInt(answer) === arr[i][1]) {
questionsRight.push(arr[i][0]);
htmlRight += "<li>" + questionsRight[right] + "</li>";
console.log(questionsRight[right]);
right += 1;
}
else {
questionsWrong.push(arr[i][0]);
htmlWrong += "<li>" + questionsWrong[wrong] + "</li>";
console.log(questionsWrong[wrong]);
wrong += 1;
}
}
htmlRight += "</ol> </div>";
htmlWrong += "</ol> </div>";
console.log(htmlRight);
console.log(htmlWrong);
html = "You got " + right + " out of " + questionsAsked + " answered correct.";
print(html);
print (htmlRight);
print (htmlWrong);
1 Answer
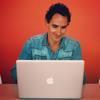
Remi Vledder
14,144 PointsHi Marvin,
It is because the print function will only convert one instance to HTML. So you could do (amongst others as always) two things,
Alter the print function to loop through the amount of times you'd like to print something.
-Or-
Alter your code a bit and use the build list function that David also mentions so that you will only use the print function once.
This is imho recommended since you will separate the concerns, and will keep your code DRY since you don't have to duplicate the HTML for the ordered list (<ol></ol>
):
var arr = [
["Hur lång är Marvin Benno? ", 2],
["Är Putin och McGregor vänner? ", 2],
["Hur många dagar finns på ett år? ", 2],
["Vart fiskar Marvin gädda? ", 2],
["1?", 2]
];
var questionsAsked = 0;
var wrong = 0;
var right = 0;
var answer;
var html;
var htmlRight;
var htmlWrong;
var questionsRight = [];
var questionsWrong = [];
function print(message){
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr) {
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i++) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
for (var i = 0; i < arr.length; i++) {
answer = prompt(arr[i][0]);
questionsAsked += 1;
if (parseInt(answer) === arr[i][1]) {
questionsRight.push(arr[i][0]);
htmlRight += questionsRight[right];
// console.log(questionsRight[right]);
right += 1;
}
else {
questionsWrong.push(arr[i][0]);
htmlWrong += questionsWrong[wrong];
// console.log(questionsWrong[wrong]);
wrong += 1;
}
}
html = "You got " + right + " out of " + questionsAsked + " answered correct.";
html += '<h1>You got these questions right </h1>';
html += buildList(questionsRight);
html += '<h1> You got these questions wrong </h1>';
html += buildList(questionsWrong);
print(html);
Here's a fiddle for you to play around with to see the end result: https://jsfiddle.net/Spindle/L8socu3v/
Good luck and happy coding!