Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial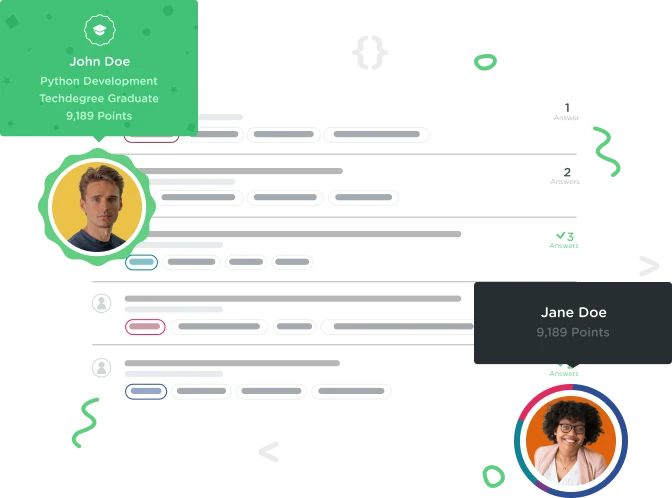

Michael Russell
1,575 PointsQuestion regarding previous "Frustration" task.
Apologies, but this question is actually about the previous "Frustration" task challenge. Treehouse's code reader said they I did things correctly when I used the following code:
class Liar (list): def init(self): self.slots = []
def add (self, item):
self.slots.append(item)
def __len__(self):
return len(self.slots + 2
However, i didn't end up using the super() function for this task, and the instructions at the beginning said I'd probably need to. I asked about this in get help forum for the task, but their explanation hasn't really helped, and every other solution I've tried has not worked. Could anyone here perhaps explain or show me an example of how super() would be applied to this particular problem? I would appreciate any feedback. Thank you.
Michael
1 Answer
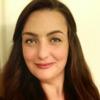
Jennifer Nordell
Treehouse TeacherHi there, Michael Russell!
The super()
can be tough to get your head around. The Liar
is the child class of list
. It inherits things from list
like the append
method etc. It also inherits the len()
that you've used so many times before. When you type len()
it runs the __len__
method of that class. You might have heard that Python is an object-oriented language. Even if it's not immediately obvious, a list
is an object.
So this challenge was asking you to override the __len__
function so that any time you do a len()
on anything that happens to be an instance of Liar
it will... lie
So here is my solution along with something you can actually test in a workspace or on your machine. Dealer's choice
class Liar(list):
def __len__(self):
return super().__len__() + 35
wrong_size = Liar(["apples", "bananas", "oranges"])
print(len(wrong_size))
This overrides the standard __len__
method that is called every time you use a len()
. So it goes out to the parent class and runs its __len__
method and then adds 35 to it. Although I sent in three things to that list, the output is 38. Because it took the real length and added 35 to it.
Hope this helps!