Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial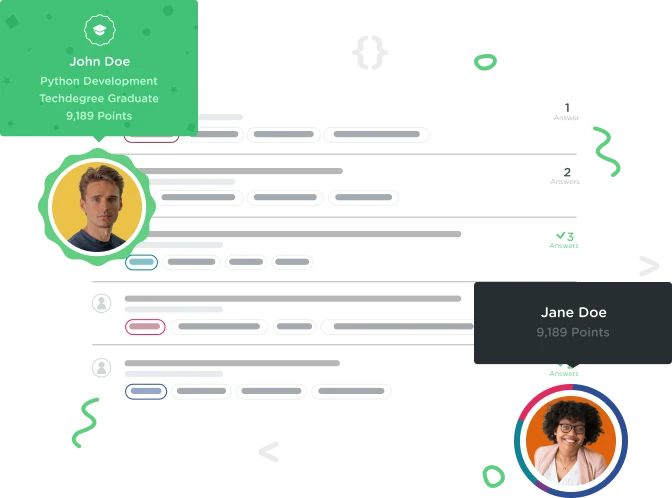

Moses Ong
889 PointsQuestion seems easy at first...
Can't seem to get the first 4 values and the last 4 values. Tried researching on start, stop step but I still don't understand :[
def first_and_last4(list):
final_list = list[0:]
return final_list
1 Answer

andren
28,558 PointsYou cannot get the first four and the last four with a single slice, but you can pretty easily accomplish the task by adding together two sliced strings. Like this:
def first_and_last_4(list):
first_part = list[:4] # Start at 0 (default when no start is specified) and end at 4
second_part = list[-4:] # Start at -4 and go until the end (default when no end is specified)
final_part = first_part + second_part # Concatenate the two resulting strings together
return final_part # return the resulting string
I made the function more verbose than it needed to be and included comments in order to make it easier to understand.
Looking at your code I notice that you have removed or changed the first_4
function you wrote during task 1. When doing multi-part challenges you need to keep the code from all of the parts around in order to pass the challenge, so removing that function will keep your code from passing. You also have a typo in the name of the function, it should be called first_and_last_4 not first_and_last4.
Here is an example of code that will pass task 2:
def first_4(list):
return list[:4]
def first_and_last_4(list):
return list[:4] + list[-4:] # Slice and concatenate the strings, then return them
I added the first_4
function back and wrote an example of first_and_last_4
that is more succinct than the example I showed earlier, but that ultimately does the same thing.