Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial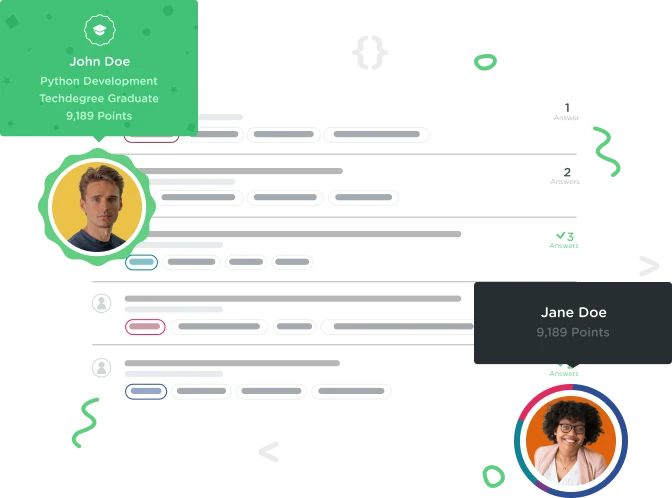
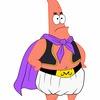
<noob />
17,062 PointsQuestions about a method.
what this method actually does?:
public int distanceTo(Point point)
{
return distanceTo(point.x, point.y);
}
how it computes the distance if we only pass 1 point? I know we overload this method :
public int distanceTo(int x, int y)
{
int xDiff = this.x - x;
int yDiff = this.y - y;
int xDiffSquared = xDiff * xDiff;
int yDiffSquared = yDiff * yDiff;
//casting the result of math.Sqrt to int(it returns double)
return (int)Math.Sqrt(xDiffSquared + yDiffSquared);
}
**UPDATE* FROM HERE: i have another question about this method which is the main reason i open this thread:
public bool inRangeOf(MapLocation location, int range)
{
return distanceTo(location) <= range;
}
another question i have is what happend if the distance is less than the range? My thoughts are this but i dont 100% sure im right on this: If the distance is less than the passed in range, for example the result of distanceTo(location) is 3 and the passed in range parameter is 5, then if 3< 5 , that means that means we are in range because The range is 5 and because of that we see the computed location(3) because he is in the range? >> please tell me if am right about this.
if the result of the distanceTo(location) is 5 and the range is 3 >> then 5<3 isnt right because the location is 5 and the range "couldn't see" lets say above 3.
2 Answers
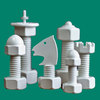
Steven Parker
231,275 PointsYou only need to pass one Point because a "Point" object contains both the X and Y values. And the object that the method is called on contains it's own coordinates, so this is computing the distance between the current object ("this") and the point that you are passing in.
The method then uses the well-known "Pythagorean theorem" to compute the distance between two points.
And finally, the overload lets you call "distanceTo" with a single point argument, but it then separates it into X and Y and calls the other version of itself that takes the coordinates as two arguments.
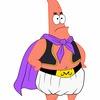
<noob />
17,062 Pointscan u go through an example of what u tried to explain to me in the first question?
lets say I have a Point which is (3,5) this point is passed to the overloaded distanceTo method, but where is the "this" object ur talking about? i get what ur saying but im not 100% sure...
return distanceTo(location) <= range;
it just take 1 point in this method..
i suspect we see the "this" object on this line:
if(invader.isActive && _location.inRangeOf(invader.Location, _range))
{
//cool code here
}
the _location variable is used as the second point which u refer as the "this"(the current object) that we are calculating the distance between the current object and the (3,5) in this example ?
Thanks again for taking ur time and answer my questions
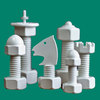
Steven Parker
231,275 PointsThat's right. The "this" always refers to the object that you call the method on.
<noob />
17,062 Points<noob />
17,062 Points@Hey steven i updated my question can u please answer it again?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsIt sounds like you understand that correctly. When the distance is less than or equal to the range, then "inRangeOf" will return a "true" result.