Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial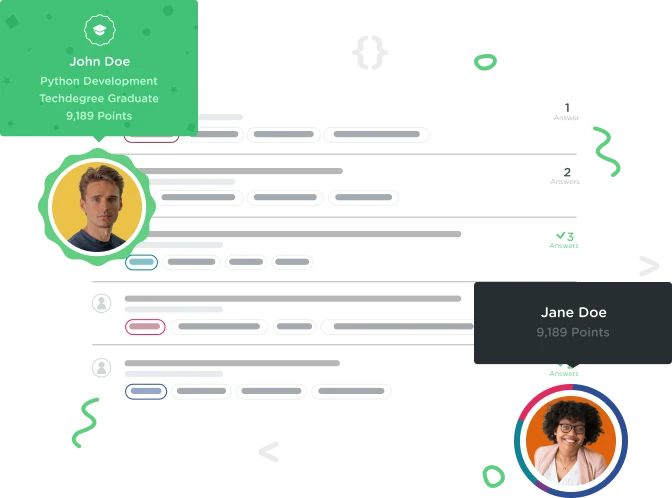

Elena Chen
1,503 PointsQuestions about attributes and self
Hi I have a few questions as below, appreciate if anyone can help! Thank you!
What does "pass" mean? Class Thief pass
We can use self to represent current instance, but I'm confused by self and the actual instances. For example, if a methods is called by a class, Thief.pocketpick(), the error message would be "pickpocket() missing 1 required positional argument: 'self'", why don't we use Thief.pocketpick(slef) but Thief.pocketpick(kenneth) instead?
Print("Called by {}".format(self)) Returned: Called by <characters. Thief object at 0x7f..... Why not return "Called by keneeth" if self represents keneeth????
Kenneth said instances are responsible for their own attribute values, if you change the sneaky attributes just on the kenneth instance, that will not change the class's attributes(Video: Let's Make a Class! 03:41)
class Thief:
sneaky = True
keneeth = Thief()
kenneth.sneaky = false
Thief.sneaky = True
However, it will change the methods If you change kenneth.sneaky = false, both kenneth.pickpocket() and Thief.pickpocket(kenneth) will return False. Why is that so?
1 Answer
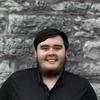
Michael Hulet
47,913 PointsIn Python, pass
means "Something will be here in the future, but not right now. Just leave this empty and continue". You'll generally never see this in shipping code, but it's nice when you're building something so that you can build out some parts of your app before others, but still be able to check syntax and such along the way
In Python, self
represents a single instance of your class (specifically, the one you're working on), and not the class itself. In fact, calling it self
is actually just a convention, and you can name it whatever you'd like. For example, this is totally fine:
class Printer:
def __repr__(this):
print(this.string)
def __init__(this, string):
this.string = string
def dump(this):
print(this.string)
Note how in the above example, I used the word this
instead of self
, but if you paste it into a file and run it, it still works just fine.
Now, let's say that I want to call the dump
function. I can do this in either of 2 ways:
Printer("Something").dump()
Printer.dump(Printer("Something"))
Both of these examples will print the string "Something"
to your console. In the first example, Python knows what to use for self
because you called dump
on a specific instance, so Python passes that instance to dump
as the first parameter. In the second, you've called the dump
function on the Printer
class and not on a specific instance, so you have to provide the value for self
yourself by manually passing the function an instance of the Printer
class. Both of these lines are exactly equivalent, but the 2nd is generally considered bad style, so you should generally always use the 1st way of doing it
I know this is counterintuitive, but Python doesn't actually know/care what you name your variables. This is pretty low-level and isn't really something you ever need to think about in your day-to-day programming, but when you make a variable, what Python sees is "I can find a bit of data at this address in memory", and not actually the specific name of your variable
If you print
out an instance of an object, Python needs to convert it to a string. By default, every object converts itself to a string in the format of <modulename.ClassName object at 0xfffff>
where modulename
is name of the file where the class is declared, ClassName
is the name of the class, and 0xfffff
is the memory address of that instance. If you'd like to change this behavior, you can provide a custom implementation of either the special __str__
or __repr__
functions for your class, like I did for Printer
in the example above
The answer to your last question is basically the same as the answer to your second question. Those are 2 different ways of doing the exact same thing. Calling kenneth.pickpocket()
is exactly the same as calling Thief.pickpocket(kenneth)
. In the first way, Python knows what instance to provide for self
because you called the pickpocket
method on that instance, and in the 2nd, you're manually providing the instance for self
yourself
Elena Chen
1,503 PointsElena Chen
1,503 PointsThank you very much Michael for your detailed answers which are very helpful!!